How to Fit Grid Models to Data¶
In this notebook, we show how to use the PICASO
-formatted grid models to interpret data. We will use the results of the JWST Transiting Exoplanet Community Early Release Science Team’s first look analysis of WASP-39 b.
Helpful knowledge before running this notebook:
Need to do before running this notebook
In order to use this notebook you will have to:
[1]:
import numpy as np
import os
import picaso.justdoit as jdi
import picaso.justplotit as jpi
import picaso.analyze as lyz
jpi.output_notebook()
Define Paths To Data and Models¶
You should have four folders in your model_dir
:
RCTE_cloud_free/
: 192 modelsRCTE_cloudy/
: 3840 modelsphotochem_cloud_free/
: 116 modelsphotochem_cloudy/
: 580 models
[2]:
#should have sub folders similar to above
#agnostic to where it is, just make sure you point to the right data file
model_dir = "/data2/models/WASP-39B/xarray/"
#downloaded and unzipped from Zenodo
data_dir = '/data2/observations/WASP-39b/ZENODO/TRANSMISSION_SPECTRA_DATA/'
#for this tutorial let's grab the firely reduction
data_file = os.path.join(data_dir,"FIREFLY_REDUCTION.txt")
wlgrid_center,rprs_data2,wlgrid_width, e_rprs2 = np.loadtxt(data_file,usecols=[0,1,2,3],unpack=True,skiprows=1)
#for now, we are only going to fit 3-5 um
wh = np.where(wlgrid_center < 3.0)
wlgrid_center = np.delete(wlgrid_center,wh[0])
wlgrid_width = np.delete(wlgrid_width,wh[0])
rprs_data2 = np.delete(rprs_data2,wh[0])
e_rprs2 = np.delete(e_rprs2,wh[0])
reduction_name = "Firefly"
[3]:
f=jpi.plot_errorbar(wlgrid_center, rprs_data2,e_rprs2, plot_type='matplotlib',
plot_kwargs={'ylabel':r'(R$_p$/R$_*$)$^2$'})#plot_type='bokeh' also available
#jpi.show(f) #if using bokeh (note if using bokeh need those key words (e.g. y_axis_label instead of ylabel))
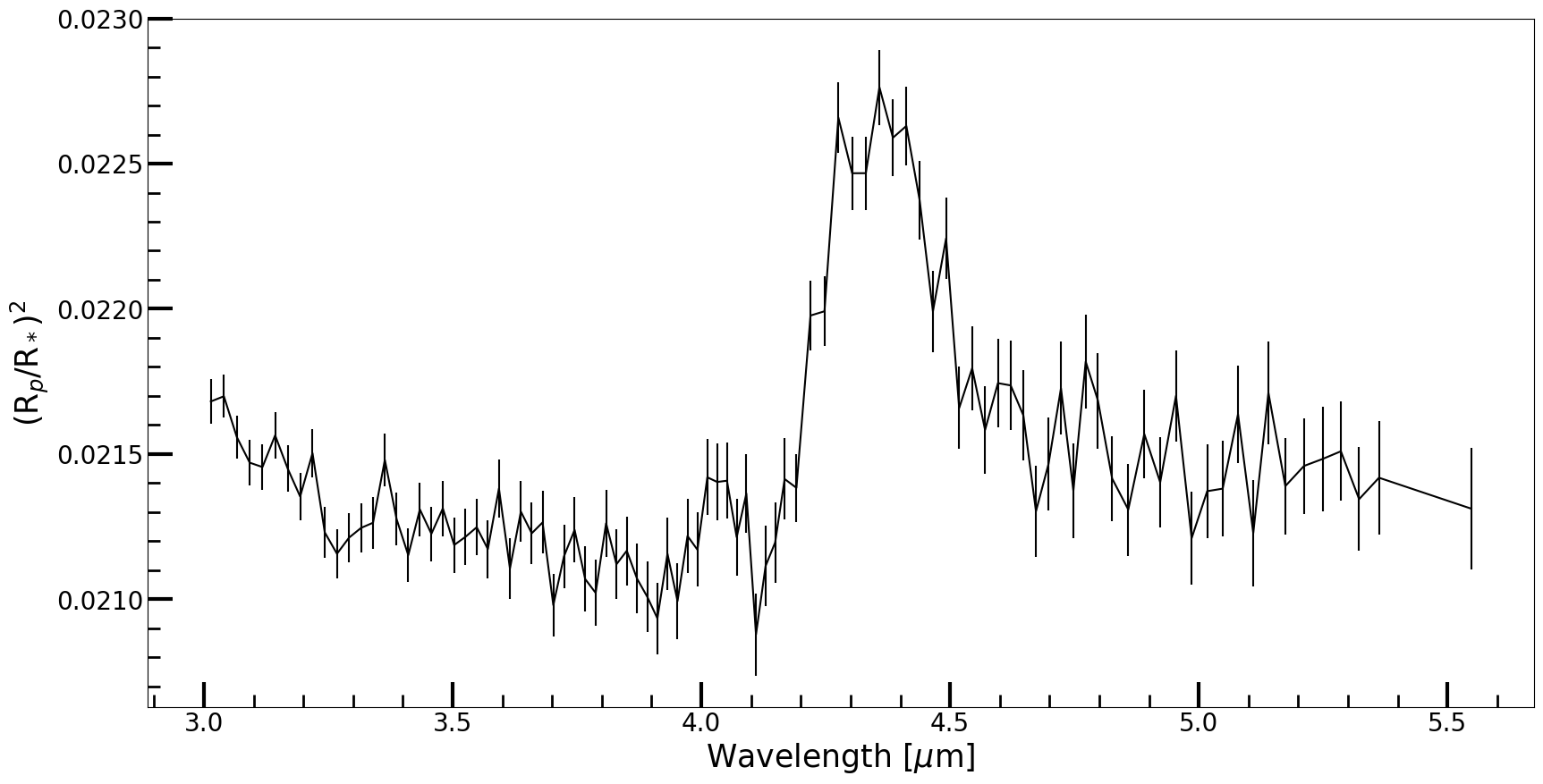
Add Available Grids to Test¶
First step will be to load your first grid into the GridFitter
class. You can do this easily by supplying the function a directory location, and grid name (grid_name
).
The only purpose of grid_name
is in case you add more grids to your GridFitter
function, it will be easy to keep track of what parameters go with what grid.
[4]:
grid_name = "picaso_cld_free"
location = os.path.join(model_dir,"RCTE_cloud_free")
fitter = lyz.GridFitter(grid_name,location, verbose=True)
Total number of models in grid is 192
For tint in planet_params grid is: [100. 200. 300.]
For heat_redis in planet_params grid is: [0.4 0.5]
For mh in planet_params grid is: [ 0.1 0.316 1. 3.162 10. 31.623 50.119 100. ]
For cto in planet_params grid is: [0.229 0.458 0.687 0.916]
This shows you what parameters the grid was created over
[5]:
fitter.grid_params['picaso_cld_free']['planet_params'].keys()
[5]:
dict_keys(['tint', 'heat_redis', 'mh', 'cto'])
[6]:
location = os.path.join(model_dir,"RCTE_cloudy")
fitter.add_grid('picaso_cldy', location)#
Total number of models in grid is 3840
For tint in planet_params grid is: [100. 200. 300.]
For heat_redis in planet_params grid is: [0.4 0.5]
For logkzz in planet_params grid is: [ 5. 7. 9. 11.]
For mh in planet_params grid is: [ 0.1 0.316 1. 3.162 10. 31.623 50.119 100. ]
For cto in planet_params grid is: [0.229 0.458 0.687 0.916]
For fsed in cld_params grid is: [ 0.6 1. 3. 6. 10. ]
Explore the parameters of the grid¶
You can see what grids you have loaded
[7]:
fitter.grids #what grids exist
[7]:
['picaso_cld_free', 'picaso_cldy']
You can also see what the top level information about your grid is
[8]:
fitter.overview['picaso_cld_free']#top level info from the attrs
[8]:
{'planet_params': {'rp': 1.279,
'mp': 0.28,
'tint': array([100., 200., 300.]),
'heat_redis': array([0.4, 0.5]),
'p_reference': 10.0,
'logkzz': 'Not used in grid.',
'mh': array([ 0.1 , 0.316, 1. , 3.162, 10. , 31.623, 50.119,
100. ]),
'cto': array([0.229, 0.458, 0.687, 0.916]),
'p_quench': 'Not used in grid.',
'rainout': 'Not used in grid.',
'teff': nan,
'logg': nan,
'm_length': nan},
'stellar_params': {'rs': 0.932,
'logg': 4.38933,
'steff': 5326.6,
'feh': -0.03,
'ms': 0.913},
'cld_params': {'opd': 'Not used in grid.',
'ssa': 'Not used in grid.',
'asy': 'Not used in grid.',
'p_cloud': 'Not used in grid.',
'haze_eff': nan,
'fsed': 'Not used in grid.'},
'num_params': 4}
The full list of planet parameters can also be cross referenced against the full list of file names so you can easily plot of different models.
[9]:
print(fitter.grid_params['picaso_cld_free']['planet_params']['tint'][0],
#this full list can be cross referened against the file list
fitter.list_of_files['picaso_cld_free'][0])
#in this case we can verify against the filename
100.0 /data2/models/WASP-39B/xarray/RCTE_cloud_free/profile_eq_planet_100_grav_4.5_mh_+1.0_CO_2.0_sm_0.0486_v_0.4_.nc
Add Datasets to Explore¶
Though the models are interesting, what we are really after is which is most representative of the data. So now let’s add some datasets to explore.
[10]:
fitter.add_data('firefly',wlgrid_center, wlgrid_width, rprs_data2, e_rprs2)
Compute \(\chi_{red}^2\)/N and Retrieve Single Best Fit¶
In this analysis we used the reduced chi sq per data point as a metric to fit the grid. This fitter function will go through your whole grid and compute cross reference the chi sq compared to your data.
[11]:
fitter.fit_grid('picaso_cld_free','firefly')
fitter.fit_grid('picaso_cldy','firefly')
Now that we have accumulated results let’s turn this into a dictionary to easily see what we’ve done
[12]:
out = fitter.as_dict()#allows you to easily grab data
out.keys()
[12]:
dict_keys(['list_of_files', 'spectra_w_offset', 'rank_order', 'grid_params', 'offsets', 'chi_sqs', 'posteriors'])
We are most interested in the models with the best reduced chi sq. We can use our ranked order to get the models that best fit the data.
[13]:
### Use rank order to get the top best fit or other parameters
#top 5 best fit models metallicities for the cloud free grid
print("cld free",np.array(out['grid_params']['picaso_cld_free']['planet_params']['mh']
)[out['rank_order']['picaso_cld_free']['firefly']][0:5])
#top 5 best fit models metallicities for the cloudy grid
print("cldy",np.array(out['grid_params']['picaso_cldy']['planet_params']['mh']
)[out['rank_order']['picaso_cldy']['firefly']][0:5])
cld free [100. 100. 100. 100. 100.]
cldy [ 3.162 10. 10. 10. 10. ]
Interesting! We are already seeing interesting information. Without clouds our model predicts higher metallicity than when we add clouds. Let’s look at the associated chi square values.
[14]:
#top 5 best fit chi sqs for the cloud free grid
print("cld free", np.array(out['chi_sqs']['picaso_cld_free']['firefly']
)[out['rank_order']['picaso_cld_free']['firefly']][0:5])
#top 5 best fit chi sq for the cloudy grid
print("cldy", np.array(out['chi_sqs']['picaso_cldy']['firefly']
)[out['rank_order']['picaso_cldy']['firefly']][0:5])
cld free [1.91987567 1.92849109 1.9556125 2.00119925 2.01856747]
cldy [1.32818737 1.37253841 1.37564302 1.39244227 1.39555045]
The cloudy grid is giving lower chi square giving us clues that this planet likely has clouds affecting the spectrum.
Analyze Single Best Fits¶
Let’s analyze the single best fits in order to compare the spectrum with the data
[15]:
fig,ax = fitter.plot_best_fit(['picaso_cld_free','picaso_cldy'],'firefly')
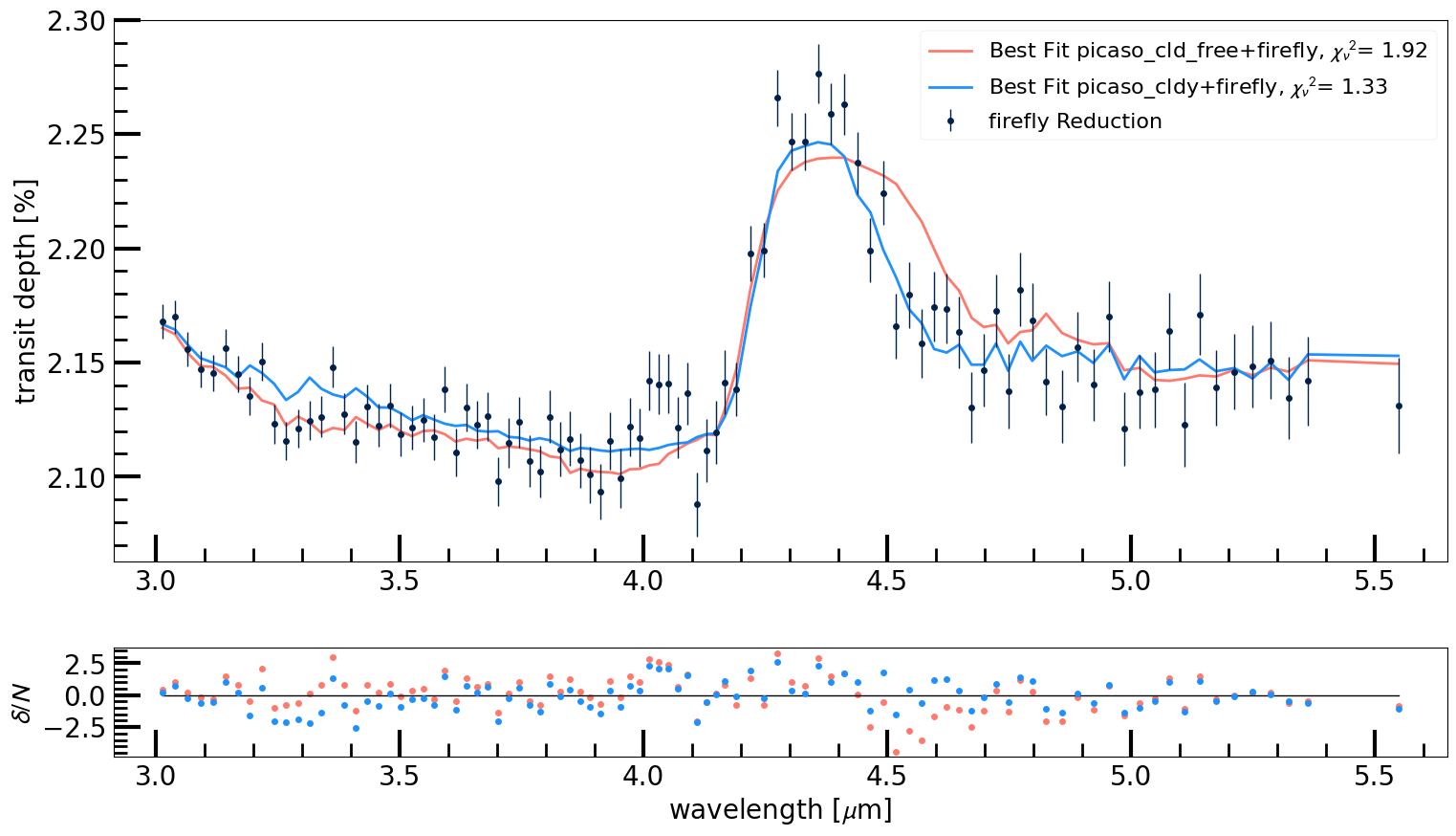
By-eye, our cloudy grid is giving a much better representation of the data. Let’s look at what physical parameters are associated with this.
[16]:
best_fit = fitter.print_best_fit('picaso_cldy','firefly')
tint=100.0
heat_redis=0.5
logkzz=9.0
mh=3.162
cto=0.458
fsed=0.6
You can see these same parameters reported in original Nature paper: https://arxiv.org/pdf/2208.11692.pdf
Estimated Posteriors¶
It is also helpful to get an idea of what the probability is for each grid parameter in your model. This will give you a better representation of degeneracies that exist with your data and each of your physical parameters.
[17]:
posterior_chance_dict, fig = fitter.plot_chi_posteriors(['picaso_cldy', 'picaso_cld_free'],
'firefly', max_row=3, max_col=2, input_parameters='all')
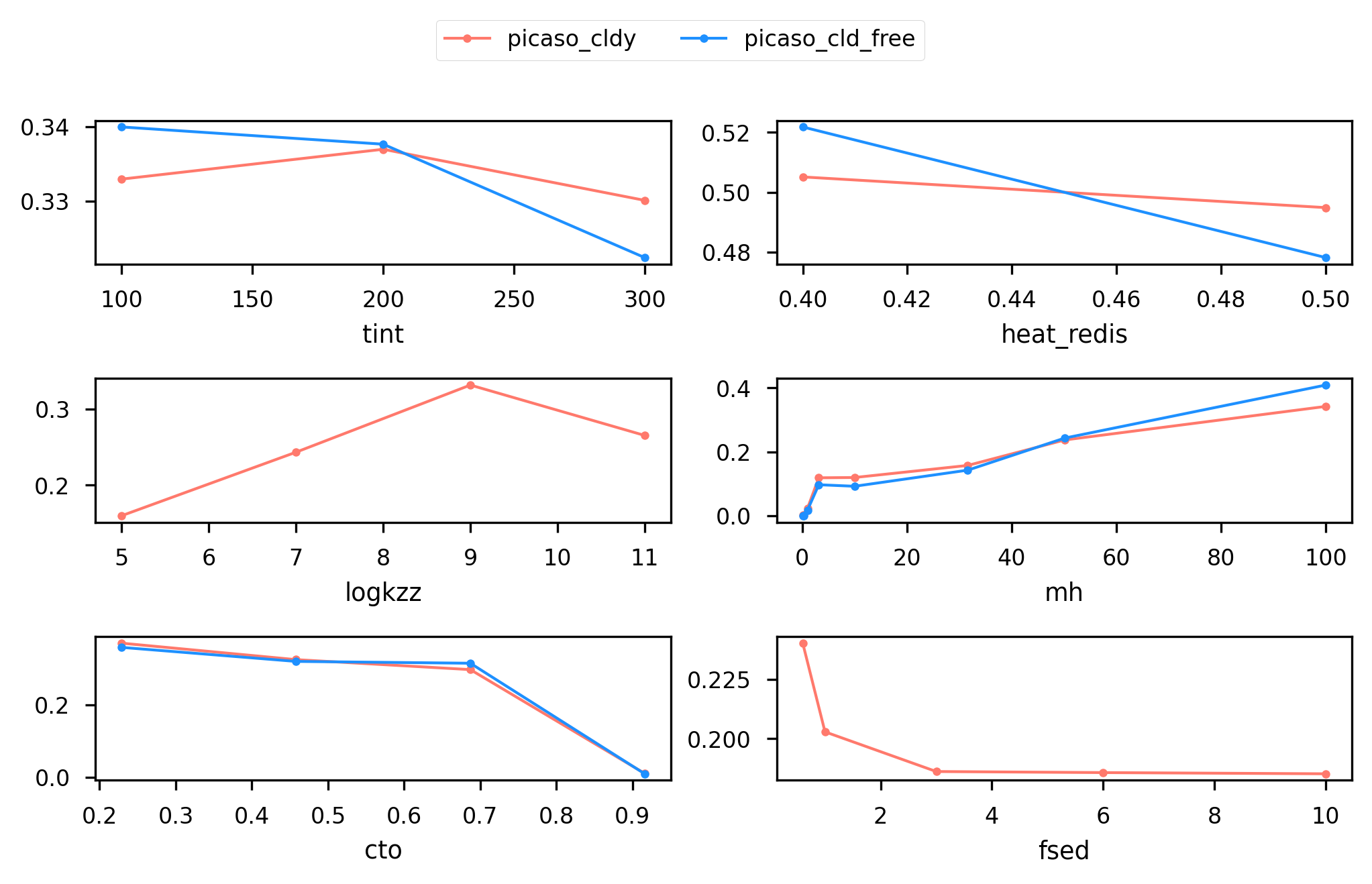
[18]:
posterior_chance_dict
[18]:
{'picaso_cldy': {'tint': {'100.0'},
'heat_redis': {'0.4'},
'logkzz': {'9.0'},
'mh': {'100.0'},
'cto': {'0.229'},
'fsed': {'0.6'}},
'picaso_cld_free': {'tint': {'100.0'},
'heat_redis': {'0.4'},
'logkzz': {'9.0'},
'mh': {'100.0'},
'cto': {'0.229'},
'fsed': {'0.6'}}}
What can you take away from this plot? 1. Cloudy models reduce the number of models that can be fit to the data with high metallicity 2. Internal temperature cannot be constrained by the data 3. C/O ratios greater than ~0.8 can be ruled out by the data
Interpret Best Fit¶
Now that we are happy with the best-fitting model, we can load in that data and post process some plots in order to gain better understanding of our results.
We can use PICASO
’s xarray
loader to quickly load in one of our models.
[19]:
#grab top model
top_model_file = np.array(out['list_of_files']['picaso_cldy']
)[out['rank_order']['picaso_cldy']['firefly']][0]
xr_usr = jdi.xr.load_dataset(top_model_file)
#take a look at the Xarray file
xr_usr
[19]:
<xarray.Dataset> Size: 606kB Dimensions: (pressure: 91, wavelength: 8988, pressure_cld: 90, wno_cld: 196) Coordinates: * pressure (pressure) float64 728B 1e-06 ... 1.8e+03 * wavelength (wavelength) float64 72kB 6.0 ... 0.3001 * pressure_cld (pressure_cld) float64 720B 1.118e-06 ...... * wno_cld (wno_cld) float64 2kB 43.95 ... 3.731e+04 Data variables: (12/54) opd (pressure_cld, wno_cld) float64 141kB 1.1... ssa (pressure_cld, wno_cld) float64 141kB 4.6... asy (pressure_cld, wno_cld) float64 141kB 9.4... condensate_mmr_Na2S (pressure_cld) float64 720B 0.0 0.0 ... 0.0 condensate_plus_gas_mmr_Na2S (pressure_cld) float64 720B 0.0001185 ...... mean_particle_r_Na2S (pressure_cld) float64 720B 0.0 0.0 ... 0.0 ... ... OCS (pressure) float64 728B 8.033e-09 ... 7.5... Li (pressure) float64 728B 7.679e-12 ... 6.1... LiOH (pressure) float64 728B 4.33e-10 ... 2.3e-09 LiH (pressure) float64 728B 2.283e-15 ... 7.3... LiCl (pressure) float64 728B 6.089e-09 ... 4.2... transit_depth (wavelength) float64 72kB 0.02463 ... 0.0... Attributes: author: Sarah E. Moran contact: semoran@lpl.arizona.edu code: {"spectrum": "PICASO", "climate": "PICASO", "chemistry":... doi: Mukherjee et al., submitted; Batalha et al., in prep planet_params: {"rp": {"value": 1.279, "unit": "jupiterRad"}, "mp": {"v... stellar_params: {"rs": {"value": 0.932, "unit": "solRad"}, "logg": 4.389... orbit_params: {"sma": 0.0486} atmo_params: {"mh": 0.5, "cto": 1.0} cld_params: {"fsed": 0.6, "species": "Na2S, MnS, MgSiO3"}
- pressure: 91
- wavelength: 8988
- pressure_cld: 90
- wno_cld: 196
- pressure(pressure)float641e-06 1.267e-06 ... 1.8e+03
- units :
- bar
array([1.000000e-06, 1.267174e-06, 1.605731e-06, 2.034741e-06, 2.578371e-06, 3.267246e-06, 4.140170e-06, 5.246317e-06, 6.647998e-06, 8.424172e-06, 1.067489e-05, 1.352695e-05, 1.714101e-05, 2.172064e-05, 2.752384e-05, 3.487751e-05, 4.419588e-05, 5.600388e-05, 7.096668e-05, 8.992716e-05, 1.139534e-04, 1.443988e-04, 1.829785e-04, 2.318656e-04, 2.938141e-04, 3.723137e-04, 4.717864e-04, 5.978356e-04, 7.575619e-04, 9.599630e-04, 1.216440e-03, 1.541442e-03, 1.953276e-03, 2.475141e-03, 3.136435e-03, 3.974410e-03, 5.036270e-03, 6.381832e-03, 8.086894e-03, 1.024750e-02, 1.298537e-02, 1.645473e-02, 2.085101e-02, 2.642187e-02, 3.348111e-02, 4.242641e-02, 5.376165e-02, 6.812539e-02, 8.632674e-02, 1.093910e-01, 1.386175e-01, 1.756525e-01, 2.225824e-01, 2.820507e-01, 3.574074e-01, 4.528974e-01, 5.739000e-01, 7.272313e-01, 9.215289e-01, 1.167738e+00, 1.479727e+00, 1.875072e+00, 2.376044e+00, 3.010861e+00, 3.815286e+00, 4.834633e+00, 6.126322e+00, 7.763118e+00, 9.837224e+00, 1.246548e+01, 1.579593e+01, 2.001620e+01, 2.536402e+01, 3.214063e+01, 4.072778e+01, 5.160920e+01, 6.539785e+01, 8.287047e+01, 1.050113e+02, 1.330677e+02, 1.686199e+02, 2.136708e+02, 2.707582e+02, 3.430978e+02, 4.347648e+02, 5.509227e+02, 6.981151e+02, 8.846336e+02, 1.120985e+03, 1.420483e+03, 1.800000e+03])
- wavelength(wavelength)float646.0 5.998 5.996 ... 0.3002 0.3001
- units :
- micron
array([5.999505, 5.997505, 5.995506, ..., 0.300251, 0.300151, 0.300051])
- pressure_cld(pressure_cld)float641.118e-06 1.383e-06 ... 146.3 180.9
- units :
- bar
array([1.118306e-06, 1.382912e-06, 1.710126e-06, 2.114763e-06, 2.615143e-06, 3.233919e-06, 3.999105e-06, 4.945343e-06, 6.115474e-06, 7.562472e-06, 9.351848e-06, 1.156461e-05, 1.430095e-05, 1.768473e-05, 2.186916e-05, 2.704368e-05, 3.344255e-05, 4.135548e-05, 5.114071e-05, 6.324125e-05, 7.820492e-05, 9.670920e-05, 1.195918e-04, 1.478887e-04, 1.828811e-04, 2.261530e-04, 2.796637e-04, 3.458357e-04, 4.276647e-04, 5.288556e-04, 6.539895e-04, 8.087316e-04, 1.000088e-03, 1.236721e-03, 1.529345e-03, 1.891207e-03, 2.338691e-03, 2.892054e-03, 3.576351e-03, 4.422560e-03, 5.468994e-03, 6.763027e-03, 8.363244e-03, 1.034209e-02, 1.278916e-02, 1.581524e-02, 1.955732e-02, 2.418483e-02, 2.990727e-02, 3.698371e-02, 4.573452e-02, 5.655588e-02, 6.993771e-02, 8.648586e-02, 1.069495e-01, 1.322551e-01, 1.635483e-01, 2.022459e-01, 2.500999e-01, 3.092766e-01, 3.824554e-01, 4.729491e-01, 5.848549e-01, 7.232389e-01, 8.943663e-01, 1.105985e+00, 1.367675e+00, 1.691284e+00, 2.091463e+00, 2.586329e+00, 3.198287e+00, 3.955042e+00, 4.890855e+00, 6.048093e+00, 7.479148e+00, 9.248808e+00, 1.143719e+01, 1.414338e+01, 1.748988e+01, 2.162820e+01, 2.674571e+01, 3.307408e+01, 4.089982e+01, 5.057724e+01, 6.254445e+01, 7.734325e+01, 9.564364e+01, 1.182741e+02, 1.462593e+02, 1.808661e+02])
- wno_cld(wno_cld)float6443.95 72.05 ... 3.484e+04 3.731e+04
- units :
- cm**(-1)
array([ 43.950055, 72.049743, 102.299697, 135.69994 , 185.601069, 236.89946 , 279.696809, 336.700337, 402.592697, 467.398925, 515.942627, 550.055006, 588.997526, 633.834062, 686.106346, 735.94348 , 782.044264, 834.306691, 885.582713, 935.278713, 990.982063, 1036.484245, 1069.74754 , 1111.728738, 1153.003574, 1198.322349, 1238.543473, 1265.182186, 1296.344309, 1333.688984, 1372.872048, 1409.641951, 1454.968718, 1500.60024 , 1534.919417, 1588.057805, 1649.076517, 1695.202577, 1736.412572, 1794.687724, 1859.081614, 1899.335233, 1942.124684, 2000. , 2048.340844, 2071.680133, 2085.070892, 2105.263158, 2127.659574, 2145.002145, 2171.55266 , 2220.248668, 2275.312856, 2325.040688, 2379.819134, 2454.590083, 2532.286655, 2598.077423, 2667.377967, 2730.748225, 2787.844996, 2846.569883, 2908.66783 , 2973.535534, 3022.974607, 3059.039462, 3095.017023, 3131.850924, 3168.567807, 3207.184092, 3241.491086, 3273.322422, 3317.850033, 3372.681282, 3437.607425, 3512.469266, 3588.087549, 3663.003663, 3736.920777, 3812.428517, 3885.003885, 3957.261575, 4029.008864, 4103.405827, 4177.10944 , 4248.08836 , 4321.521175, 4393.673111, 4464.285714, 4526.935265, 4580.852038, 4623.208507, 4655.493482, 4679.457183, 4697.040864, 4730.368969, 4782.400765, 4830.917874, 4878.04878 , 4926.108374, 4975.124378, 5045.408678, 5136.106831, 5219.206681, 5293.806247, 5367.686527, 5443.658138, 5518.763797, 5592.841163, 5662.514156, 5747.126437, 5837.711617, 5910.165485, 5970.149254, 6024.096386, 6075.334143, 6146.2815 , 6234.413965, 6313.131313, 6381.620932, 6443.298969, 6501.950585, 6565.988181, 6640.106242, 6720.430108, 6798.096533, 6887.052342, 6973.500697, 7062.146893, 7142.857143, 7215.007215, 7278.020378, 7363.77025 , 7468.259895, 7587.253414, 7716.049383, 7836.990596, 7942.811755, 8058.017728, 8169.934641, 8291.873964, 8410.428932, 8554.319932, 8710.801394, 8880.994671, 9074.410163, 9259.259259, 9425.070688, 9615.384615, 9842.519685, 10040.160643, 10204.081633, 10384.215992, 10593.220339, 10741.138561, 10881.392818, 11061.946903, 11223.344557, 11402.508552, 11560.693642, 11737.089202, 11990.407674, 12165.450122, 12345.679012, 12626.262626, 12870.01287 , 13071.895425, 13297.87234 , 13531.799729, 13717.421125, 14005.602241, 14347.202296, 14771.048744, 15220.700152, 15576.323988, 15923.566879, 16207.455429, 16556.291391, 16920.473773, 17301.038062, 17699.115044, 18115.942029, 18621.973929, 19120.458891, 19762.84585 , 20618.556701, 21505.376344, 22471.910112, 23474.178404, 24509.803922, 25839.793282, 27624.309392, 29673.590504, 32051.282051, 34843.205575, 37313.432836])
- opd(pressure_cld, wno_cld)float641.149e-10 2.968e-10 ... 0.0 0.0
- units :
- unitless per layer
array([[1.14860826e-10, 2.96786772e-10, 5.63576437e-10, ..., 1.98210078e-07, 2.88519610e-07, 3.85609997e-07], [1.62365327e-10, 4.19841819e-10, 7.97121565e-10, ..., 2.75610270e-07, 4.01354921e-07, 5.36672994e-07], [2.33301461e-10, 6.03533061e-10, 1.14577262e-09, ..., 3.93276117e-07, 5.73109289e-07, 7.66935196e-07], ..., [0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00]])
- ssa(pressure_cld, wno_cld)float644.654e-12 1.308e-11 ... 0.0 0.0
- units :
- unitless
array([[4.65400889e-12, 1.30768229e-11, 2.82388746e-11, ..., 4.61270284e-04, 4.62858468e-04, 4.83625287e-04], [9.57723416e-12, 2.68902704e-11, 5.80750594e-11, ..., 9.63604388e-04, 9.67133549e-04, 1.01053757e-03], [1.95315300e-11, 5.48150761e-11, 1.18392441e-10, ..., 1.98773450e-03, 1.99450031e-03, 2.08299349e-03], ..., [0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00]])
- asy(pressure_cld, wno_cld)float649.452e-21 9.282e-19 ... 0.0 0.0
- units :
- unitless
array([[9.45159915e-21, 9.28201265e-19, 1.44487089e-16, ..., 7.54293684e-03, 7.93191190e-03, 7.56075502e-03], [1.75707335e-19, 1.49031406e-17, 1.80702048e-15, ..., 1.21775210e-02, 1.25170342e-02, 1.16695273e-02], [2.78449761e-18, 2.04096155e-16, 1.93827762e-14, ..., 1.90412861e-02, 1.90729296e-02, 1.73794772e-02], ..., [0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00]])
- condensate_mmr_Na2S(pressure_cld)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- g/g
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- condensate_plus_gas_mmr_Na2S(pressure_cld)float640.0001185 0.0001185 ... 0.0001185
- units :
- g/g
array([0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849, 0.00011849])
- mean_particle_r_Na2S(pressure_cld)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- micron
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- opd_by_gas_Na2S(pressure_cld)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- units :
- unitless
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- condensate_mmr_MnS(pressure_cld)float642.342e-08 2.7e-08 ... 0.0 0.0
- units :
- g/g
array([2.34171600e-08, 2.69953457e-08, 3.11178611e-08, 3.58690195e-08, 4.13451467e-08, 4.76570795e-08, 5.49325044e-08, 6.33185624e-08, 7.29848240e-08, 8.41267295e-08, 9.69695356e-08, 1.11772873e-07, 1.28836022e-07, 1.48503966e-07, 1.71174369e-07, 1.97305593e-07, 2.27425950e-07, 2.62144394e-07, 3.02162855e-07, 3.48290412e-07, 4.01459668e-07, 4.62745614e-07, 5.33387314e-07, 6.14812921e-07, 7.08668483e-07, 8.16851049e-07, 9.41546809e-07, 1.08527489e-06, 1.25093962e-06, 1.44189071e-06, 1.66198610e-06, 1.91566396e-06, 2.20802808e-06, 2.54494915e-06, 2.93317488e-06, 3.38041659e-06, 3.89542321e-06, 4.48807831e-06, 5.16933250e-06, 5.95047529e-06, 6.84098057e-06, 7.84403019e-06, 8.95130307e-06, 1.01332485e-05, 1.12992616e-05, 1.22461154e-05, 1.27025910e-05, 1.24196218e-05, 1.11846974e-05, 8.55211495e-06, 3.43781451e-06, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00])
- condensate_plus_gas_mmr_MnS(pressure_cld)float642.342e-08 2.7e-08 ... 2.139e-05
- units :
- g/g
array([2.34248610e-08, 2.70000161e-08, 3.11212714e-08, 3.58718228e-08, 4.13476673e-08, 4.76594919e-08, 5.49348988e-08, 6.33209800e-08, 7.29872883e-08, 8.41292618e-08, 9.69721736e-08, 1.11775684e-07, 1.28839088e-07, 1.48507374e-07, 1.71178193e-07, 1.97309906e-07, 2.27430847e-07, 2.62149999e-07, 3.02169313e-07, 3.48297896e-07, 4.01468370e-07, 4.62755732e-07, 5.33399086e-07, 6.14826689e-07, 7.08684814e-07, 8.16871004e-07, 9.41572378e-07, 1.08530975e-06, 1.25098852e-06, 1.44195761e-06, 1.66207720e-06, 1.91579580e-06, 2.20823869e-06, 2.54530939e-06, 2.93380650e-06, 3.38155476e-06, 3.89754691e-06, 4.49209770e-06, 5.17700415e-06, 5.96565366e-06, 6.87293749e-06, 7.91469609e-06, 9.10643652e-06, 1.04611448e-05, 1.19838764e-05, 1.36589951e-05, 1.54359969e-05, 1.72274281e-05, 1.89126742e-05, 2.03301656e-05, 2.12102903e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05, 2.13940909e-05])
- mean_particle_r_MnS(pressure_cld)float640.0002131 0.0002714 ... 0.0 0.0
- units :
- micron
array([2.13135194e-04, 2.71405853e-04, 3.44409253e-04, 4.36070360e-04, 5.51185035e-04, 6.95855254e-04, 8.77878399e-04, 1.10713422e-03, 1.39598614e-03, 1.75989422e-03, 2.21803586e-03, 2.79415383e-03, 3.51820588e-03, 4.42816860e-03, 5.57225744e-03, 7.01108986e-03, 8.82002316e-03, 1.10936073e-02, 1.39510343e-02, 1.75419639e-02, 2.20550479e-02, 2.77283183e-02, 3.48590808e-02, 4.38165160e-02, 5.50552541e-02, 6.91251669e-02, 8.66878962e-02, 1.08538787e-01, 1.35755676e-01, 1.69789156e-01, 2.12198398e-01, 2.64509118e-01, 3.28371004e-01, 4.05880034e-01, 4.99376315e-01, 6.10540361e-01, 7.40029607e-01, 8.87405440e-01, 1.05011167e+00, 1.22272949e+00, 1.39800282e+00, 1.56941599e+00, 1.73214437e+00, 1.88250471e+00, 2.01714001e+00, 2.13489005e+00, 2.23697106e+00, 2.32476786e+00, 2.39933160e+00, 2.46148489e+00, 2.51213507e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00])
- opd_by_gas_MnS(pressure_cld)float647.706e-05 0.0001655 ... 1.939 1.939
- units :
- unitless
array([7.70556397e-05, 1.65450823e-04, 2.67199528e-04, 3.84579063e-04, 5.20220488e-04, 6.77151856e-04, 8.58842464e-04, 1.06927094e-03, 1.31303040e-03, 1.59544932e-03, 1.92275183e-03, 2.30224581e-03, 2.74246713e-03, 3.25333101e-03, 3.84630437e-03, 4.53466783e-03, 5.33389556e-03, 6.26201603e-03, 7.33998822e-03, 8.59218481e-03, 1.00469050e-02, 1.17369594e-02, 1.37005232e-02, 1.59822293e-02, 1.86346058e-02, 2.17201637e-02, 2.53139036e-02, 2.95062209e-02, 3.44019128e-02, 4.01192599e-02, 4.68010641e-02, 5.46303719e-02, 6.38416705e-02, 7.47259313e-02, 8.76459745e-02, 1.03078831e-01, 1.21671110e-01, 1.44307227e-01, 1.72226188e-01, 2.07201269e-01, 2.51765436e-01, 3.09444161e-01, 3.85015333e-01, 4.84764270e-01, 6.16304403e-01, 7.87000739e-01, 1.00113767e+00, 1.25643711e+00, 1.53874570e+00, 1.80543583e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00, 1.93874802e+00])
- condensate_mmr_MgSiO3(pressure_cld)float643.038e-08 3.502e-08 ... 0.0 0.0
- units :
- g/g
array([3.03843330e-08, 3.50228535e-08, 4.03694976e-08, 4.65323688e-08, 5.36360736e-08, 6.18242413e-08, 7.12624274e-08, 8.21414620e-08, 9.46813072e-08, 1.09135505e-07, 1.25796303e-07, 1.45000565e-07, 1.67136580e-07, 1.92651914e-07, 2.22062459e-07, 2.55962864e-07, 2.95038559e-07, 3.40079610e-07, 3.91996700e-07, 4.51839534e-07, 5.20818070e-07, 6.00326977e-07, 6.91973840e-07, 7.97611657e-07, 9.19376310e-07, 1.05972975e-06, 1.22150976e-06, 1.40798736e-06, 1.62293293e-06, 1.87069242e-06, 2.15627526e-06, 2.48545561e-06, 2.86488912e-06, 3.30224748e-06, 3.80637351e-06, 4.38745987e-06, 5.05725498e-06, 5.82930022e-06, 6.71920282e-06, 7.74494827e-06, 8.92725380e-06, 1.02899596e-05, 1.18604570e-05, 1.36701280e-05, 1.57545966e-05, 1.81536625e-05, 2.09117216e-05, 2.40791082e-05, 2.77135193e-05, 3.18774775e-05, 3.66307250e-05, 4.20256023e-05, 4.81041768e-05, 5.49057177e-05, 6.24792325e-05, 7.09165263e-05, 8.03883263e-05, 9.11477633e-05, 1.03521246e-04, 1.17866420e-04, 1.34688188e-04, 1.54741006e-04, 1.78800230e-04, 2.07322507e-04, 2.40502629e-04, 2.78644950e-04, 3.22347642e-04, 3.72459401e-04, 4.29999902e-04, 4.96138455e-04, 5.72209142e-04, 6.59732743e-04, 7.60435675e-04, 8.76282496e-04, 1.00947022e-03, 1.16234133e-03, 1.33711460e-03, 1.53493913e-03, 1.75252044e-03, 1.96944657e-03, 2.09604106e-03, 1.70673718e-03, 1.70448491e-04, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00])
- condensate_plus_gas_mmr_MgSiO3(pressure_cld)float643.038e-08 3.502e-08 ... 0.002752
- units :
- g/g
array([3.03843335e-08, 3.50228537e-08, 4.03694978e-08, 4.65323690e-08, 5.36360738e-08, 6.18242414e-08, 7.12624276e-08, 8.21414622e-08, 9.46813073e-08, 1.09135505e-07, 1.25796303e-07, 1.45000565e-07, 1.67136580e-07, 1.92651914e-07, 2.22062459e-07, 2.55962865e-07, 2.95038559e-07, 3.40079611e-07, 3.91996701e-07, 4.51839535e-07, 5.20818071e-07, 6.00326979e-07, 6.91973842e-07, 7.97611660e-07, 9.19376314e-07, 1.05972975e-06, 1.22150977e-06, 1.40798737e-06, 1.62293294e-06, 1.87069244e-06, 2.15627530e-06, 2.48545568e-06, 2.86488925e-06, 3.30224773e-06, 3.80637402e-06, 4.38746097e-06, 5.05725742e-06, 5.82930574e-06, 6.71921543e-06, 7.74497838e-06, 8.92733130e-06, 1.02901712e-05, 1.18610289e-05, 1.36716048e-05, 1.57583572e-05, 1.81631007e-05, 2.09336170e-05, 2.41244050e-05, 2.77976176e-05, 3.20240191e-05, 3.68831052e-05, 4.24623593e-05, 4.88559851e-05, 5.61637878e-05, 6.44918323e-05, 7.39555359e-05, 8.46890834e-05, 9.68565091e-05, 1.10663452e-04, 1.26364368e-04, 1.44272630e-04, 1.64791757e-04, 1.88437100e-04, 2.15813872e-04, 2.47570368e-04, 2.84387328e-04, 3.27009230e-04, 3.76284208e-04, 4.33193538e-04, 4.98873869e-04, 5.74639412e-04, 6.62006900e-04, 7.62723505e-04, 8.78798057e-04, 1.01253543e-03, 1.16656398e-03, 1.34384000e-03, 1.54757330e-03, 1.78087337e-03, 2.04536620e-03, 2.33571253e-03, 2.61788842e-03, 2.74923079e-03, 2.75187273e-03, 2.75187273e-03, 2.75187273e-03, 2.75187273e-03, 2.75187273e-03, 2.75187273e-03, 2.75187273e-03])
- mean_particle_r_MgSiO3(pressure_cld)float640.0002671 0.0003401 ... 0.0 0.0
- units :
- micron
array([2.67086776e-04, 3.40107695e-04, 4.31590721e-04, 5.46454370e-04, 6.90708515e-04, 8.71999767e-04, 1.10009932e-03, 1.38738900e-03, 1.74935823e-03, 2.20538465e-03, 2.77949815e-03, 3.50145154e-03, 4.40878515e-03, 5.54908702e-03, 6.98277415e-03, 8.78580159e-03, 1.10525895e-02, 1.39015985e-02, 1.74821512e-02, 2.19817188e-02, 2.76365816e-02, 3.47448069e-02, 4.36785795e-02, 5.48998704e-02, 6.89772279e-02, 8.65976441e-02, 1.08586532e-01, 1.35933833e-01, 1.69977630e-01, 2.12511680e-01, 2.65445219e-01, 3.30609973e-01, 4.09931738e-01, 5.05789541e-01, 6.20699028e-01, 7.56127048e-01, 9.12021680e-01, 1.08678834e+00, 1.27628116e+00, 1.47332982e+00, 1.66944452e+00, 1.85737388e+00, 2.03268483e+00, 2.19230077e+00, 2.33346011e+00, 2.45558166e+00, 2.56053194e+00, 2.65022691e+00, 2.72601306e+00, 2.78884754e+00, 2.83974025e+00, 2.88021988e+00, 2.91200436e+00, 2.93676656e+00, 2.95590422e+00, 2.97056144e+00, 2.98164344e+00, 2.98982860e+00, 2.99564710e+00, 2.99948474e+00, 3.00179227e+00, 3.00314637e+00, 3.00402359e+00, 3.00459494e+00, 3.00473909e+00, 3.00425226e+00, 3.00302089e+00, 3.00105148e+00, 2.99838924e+00, 2.99502495e+00, 2.99092459e+00, 2.98599439e+00, 2.98006501e+00, 2.97299870e+00, 2.96460561e+00, 2.95484484e+00, 2.94336846e+00, 2.92992261e+00, 2.91420903e+00, 2.89628599e+00, 2.87604392e+00, 2.85317191e+00, 2.82699644e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, 0.00000000e+00])
- opd_by_gas_MgSiO3(pressure_cld)float649.998e-05 0.0002147 ... 4.908e+05
- units :
- unitless
array([9.99815301e-05, 2.14662430e-04, 3.46661940e-04, 4.98936661e-04, 6.74900935e-04, 8.78483593e-04, 1.11418559e-03, 1.38716823e-03, 1.70339063e-03, 2.06976507e-03, 2.49436623e-03, 2.98667489e-03, 3.55776508e-03, 4.22050052e-03, 4.98975826e-03, 5.88276888e-03, 6.91960972e-03, 8.12367360e-03, 9.52215770e-03, 1.11466921e-02, 1.30340070e-02, 1.52266957e-02, 1.77743267e-02, 2.07348730e-02, 2.41765927e-02, 2.81807689e-02, 3.28450130e-02, 3.82871273e-02, 4.46439723e-02, 5.20705522e-02, 6.07548529e-02, 7.09391684e-02, 8.29362176e-02, 9.71383765e-02, 1.14042060e-01, 1.34309826e-01, 1.58853169e-01, 1.88936914e-01, 2.26353860e-01, 2.73696473e-01, 3.34722707e-01, 4.14839155e-01, 5.21763136e-01, 6.66557747e-01, 8.65223749e-01, 1.14087684e+00, 1.52675349e+00, 2.07073254e+00, 2.84203499e+00, 3.94093120e+00, 5.51238423e+00, 7.76485986e+00, 1.09963294e+01, 1.56307429e+01, 2.22701482e+01, 3.17724602e+01, 4.53710428e+01, 6.48556482e+01, 9.28432793e+01, 1.33171240e+02, 1.91522068e+02, 2.76432817e+02, 4.00722507e+02, 5.83308802e+02, 8.51693505e+02, 1.24578407e+03, 1.82372533e+03, 2.67048321e+03, 3.91033626e+03, 5.72513444e+03, 8.38103085e+03, 1.22676919e+04, 1.79558301e+04, 2.62814918e+04, 3.84694846e+04, 5.63114003e+04, 8.24212650e+04, 1.20576698e+05, 1.76078988e+05, 2.55610254e+05, 3.63650810e+05, 4.76169543e+05, 4.90823426e+05, 4.90823426e+05, 4.90823426e+05, 4.90823426e+05, 4.90823426e+05, 4.90823426e+05, 4.90823426e+05, 4.90823426e+05])
- temperature(pressure)float64790.8 786.5 ... 2.78e+03 2.936e+03
- units :
- Kelvin
array([ 790.82084923, 786.53697574, 784.91136328, 784.78140672, 785.80502937, 787.69616889, 790.15273964, 792.90668617, 795.80114855, 798.8146028 , 801.94282526, 805.31954823, 809.03242833, 813.04018351, 817.27648447, 821.61760193, 826.06470764, 830.66892821, 835.42033834, 840.30206952, 845.31302011, 850.40258256, 855.53822879, 860.76709408, 866.16726542, 871.90401004, 878.26118486, 885.49178697, 893.83052796, 902.26461438, 910.21780963, 918.72311701, 928.78894657, 940.7737895 , 953.70910828, 967.24495373, 981.83064534, 997.45420731, 1013.58586623, 1030.53303913, 1049.09656068, 1069.78838671, 1092.04615229, 1114.40700393, 1136.79221289, 1160.27141955, 1184.0866915 , 1206.39482816, 1226.88847255, 1245.66309251, 1264.21272205, 1283.4528814 , 1303.40366665, 1323.63874629, 1343.41005859, 1362.40211391, 1380.0560323 , 1396.24796307, 1410.90084606, 1424.14701273, 1436.21356398, 1446.56253908, 1454.35461727, 1459.14779224, 1461.52955096, 1462.62250846, 1463.42257677, 1464.45313419, 1465.94458408, 1468.11472751, 1471.27197889, 1475.85108677, 1482.45642985, 1491.91387314, 1504.68173751, 1521.5077598 , 1543.45738093, 1571.76452524, 1607.79749975, 1652.90031364, 1708.13760175, 1774.65371233, 1856.06815616, 1956.69461917, 2079.6890041 , 2208.3997862 , 2342.96564453, 2483.17361798, 2629.18129923, 2780.12046721, 2935.75511848])
- e-(pressure)float642.312e-14 1.689e-14 ... 5.786e-08
- units :
- v/v
array([2.31201186e-14, 1.68906447e-14, 1.37937845e-14, 1.20292482e-14, 1.10634535e-14, 1.05941222e-14, 1.04226074e-14, 1.04436558e-14, 1.05828132e-14, 1.08355439e-14, 1.10219420e-14, 1.12004171e-14, 1.15285854e-14, 1.19954205e-14, 1.25795682e-14, 1.30908241e-14, 1.35989661e-14, 1.42188798e-14, 1.49566628e-14, 1.58156465e-14, 1.65908975e-14, 1.73305894e-14, 1.82135044e-14, 1.92929163e-14, 2.06613841e-14, 2.23338913e-14, 2.48926434e-14, 2.89363513e-14, 3.54247079e-14, 4.31316410e-14, 4.92508131e-14, 5.70123119e-14, 6.90833365e-14, 8.83614756e-14, 1.15132495e-13, 1.50268330e-13, 2.01496996e-13, 2.77029995e-13, 3.78093737e-13, 5.18915717e-13, 7.29388825e-13, 1.06415394e-12, 1.58323180e-12, 2.31612077e-12, 3.31856510e-12, 4.76910681e-12, 6.77468508e-12, 9.20708101e-12, 1.19705436e-11, 1.48781540e-11, 1.82085251e-11, 2.23425004e-11, 2.74990524e-11, 3.37992768e-11, 4.07764693e-11, 4.81577044e-11, 5.53179995e-11, 6.17994120e-11, 6.72041954e-11, 7.12377943e-11, 7.39863269e-11, 7.48683785e-11, 7.30369564e-11, 6.83338764e-11, 6.15562138e-11, 5.44847538e-11, 4.80350215e-11, 4.24851719e-11, 3.78176631e-11, 3.37422053e-11, 3.05066032e-11, 2.81302769e-11, 2.66714360e-11, 2.62151232e-11, 2.67445408e-11, 2.87260488e-11, 3.28475379e-11, 4.04152027e-11, 5.39826939e-11, 7.86186041e-11, 1.24572713e-10, 2.12813316e-10, 3.96101448e-10, 8.07463178e-10, 1.76205348e-09, 3.58286952e-09, 6.85966333e-09, 1.24621117e-08, 2.16656600e-08, 3.61000346e-08, 5.78640035e-08])
- H2(pressure)float640.835 0.835 0.835 ... 0.8396 0.8454
- units :
- v/v
array([0.83503 , 0.83503036, 0.8350308 , 0.83503121, 0.83503151, 0.83503162, 0.83503153, 0.83503127, 0.83503085, 0.83503027, 0.83502978, 0.83502941, 0.835029 , 0.83502856, 0.8350281 , 0.83502763, 0.83502716, 0.83502668, 0.83502618, 0.83502568, 0.83502579, 0.83502623, 0.83502644, 0.83502644, 0.83502619, 0.83502423, 0.83502247, 0.83502112, 0.83502025, 0.83502 , 0.83502 , 0.83502 , 0.83502 , 0.83502 , 0.83501903, 0.83501573, 0.8350157 , 0.83501914, 0.83502 , 0.83501955, 0.83501654, 0.83501616, 0.83501854, 0.83502 , 0.83501797, 0.83501495, 0.83501372, 0.83501297, 0.83501087, 0.83500914, 0.83500798, 0.83500841, 0.83501 , 0.83501 , 0.83500456, 0.83500044, 0.83499955, 0.83500136, 0.83499944, 0.83498411, 0.8349669 , 0.83495413, 0.83494402, 0.83493355, 0.83486009, 0.8347868 , 0.83471403, 0.83464298, 0.83457494, 0.83441895, 0.83426102, 0.8341103 , 0.83397009, 0.83385271, 0.83376153, 0.83367661, 0.83359373, 0.83350971, 0.83343134, 0.83341891, 0.83345428, 0.8336124 , 0.83390343, 0.83428242, 0.83419092, 0.83409026, 0.83445138, 0.83516635, 0.8366817 , 0.83957286, 0.84536945])
- H(pressure)float641.802e-09 1.333e-09 ... 0.002802
- units :
- v/v
array([1.80155600e-09, 1.33285789e-09, 1.10405849e-09, 9.75307762e-10, 9.05467276e-10, 8.72368075e-10, 8.60618761e-10, 8.59206736e-10, 8.62170404e-10, 8.68672492e-10, 8.78718345e-10, 8.97198373e-10, 9.27604693e-10, 9.69158447e-10, 1.02021706e-09, 1.07652774e-09, 1.13859244e-09, 1.20929851e-09, 1.28908800e-09, 1.37806839e-09, 1.47714110e-09, 1.58441521e-09, 1.69851662e-09, 1.82285152e-09, 1.96373022e-09, 2.13547889e-09, 2.36628876e-09, 2.69248662e-09, 3.16632769e-09, 3.71660124e-09, 4.27558872e-09, 4.98297637e-09, 6.06494068e-09, 7.76999981e-09, 1.01424316e-08, 1.33301233e-08, 1.78431324e-08, 2.42662511e-08, 3.30342392e-08, 4.53026755e-08, 6.37208496e-08, 9.27171401e-08, 1.37149039e-07, 1.99379141e-07, 2.84542353e-07, 4.07507399e-07, 5.76354825e-07, 7.78668005e-07, 1.00380085e-06, 1.24082421e-06, 1.51310264e-06, 1.84903374e-06, 2.26396647e-06, 2.75897099e-06, 3.30737963e-06, 3.88628367e-06, 4.44660442e-06, 4.95423624e-06, 5.37982301e-06, 5.71061231e-06, 5.94934309e-06, 6.04624675e-06, 5.93631569e-06, 5.60525226e-06, 5.13169011e-06, 4.62191334e-06, 4.14734617e-06, 3.73229037e-06, 3.37823769e-06, 3.08361293e-06, 2.84935946e-06, 2.67934429e-06, 2.58220384e-06, 2.57457504e-06, 2.66708848e-06, 2.89065993e-06, 3.30700601e-06, 4.02872122e-06, 5.26527231e-06, 7.41141508e-06, 1.11941672e-05, 1.80532551e-05, 3.14236456e-05, 5.93970680e-05, 1.20414952e-04, 2.30198989e-04, 4.17122353e-04, 7.18391241e-04, 1.18144976e-03, 1.85701805e-03, 2.80214309e-03])
- H+(pressure)float644.502e-38 4.502e-38 ... 1.897e-20
- units :
- v/v
array([4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 6.04050005e-38, 2.78915801e-37, 1.61951540e-36, 4.23647872e-35, 1.72871799e-33, 1.14362104e-31, 1.15187606e-29, 8.26860228e-28, 4.33598838e-26, 1.68097510e-24, 4.93630703e-23, 1.09880939e-21, 1.89720124e-20])
- H-(pressure)float641.137e-25 8.379e-26 ... 9.234e-09
- units :
- v/v
array([1.13678741e-25, 8.37873820e-26, 7.38662941e-26, 7.22711049e-26, 7.68253223e-26, 8.69370241e-26, 1.02518106e-25, 1.24011310e-25, 1.52166014e-25, 1.89118569e-25, 2.33931906e-25, 2.90567491e-25, 3.68287293e-25, 4.74811158e-25, 6.19724597e-25, 8.03789334e-25, 1.04213092e-24, 1.36332963e-24, 1.79815140e-24, 2.38818299e-24, 3.15157773e-24, 4.14309006e-24, 5.47787206e-24, 7.30479580e-24, 9.87045513e-24, 1.35370754e-23, 1.93571378e-23, 2.93248058e-23, 4.77077456e-23, 7.70585064e-23, 1.15172019e-22, 1.75834051e-22, 2.88204448e-22, 5.13766551e-22, 9.43226582e-22, 1.74153811e-21, 3.33899984e-21, 6.62489860e-21, 1.30170989e-20, 2.58146069e-20, 5.31990480e-20, 1.16035222e-19, 2.60545867e-19, 5.68052397e-19, 1.19863867e-18, 2.54185014e-18, 5.29050149e-18, 1.02546591e-17, 1.84775845e-17, 3.10905670e-17, 5.11216741e-17, 8.43776401e-17, 1.39784623e-16, 2.30149791e-16, 3.68590337e-16, 5.71544446e-16, 8.49457379e-16, 1.21003325e-15, 1.65223490e-15, 2.17126013e-15, 2.76741602e-15, 3.39083545e-15, 3.93110938e-15, 4.27942350e-15, 4.41076135e-15, 4.42751043e-15, 4.41785651e-15, 4.42936297e-15, 4.48341886e-15, 4.56958442e-15, 4.75081020e-15, 5.08550753e-15, 5.67259879e-15, 6.68130601e-15, 8.33543477e-15, 1.12066007e-14, 1.65093312e-14, 2.70618662e-14, 4.99619312e-14, 1.04312627e-13, 2.45741219e-13, 6.43254547e-13, 1.90113182e-12, 6.38416310e-12, 2.35687371e-11, 7.81951758e-11, 2.36202214e-10, 6.55950235e-10, 1.69403540e-09, 4.08178325e-09, 9.23399881e-09])
- H2-(pressure)float641.372e-36 1.232e-36 ... 1.208e-10
- units :
- v/v
array([1.37187503e-36, 1.23181459e-36, 1.24940038e-36, 1.36442800e-36, 1.58543147e-36, 1.93749706e-36, 2.45481610e-36, 3.18330932e-36, 4.19000992e-36, 5.59270396e-36, 7.91830401e-36, 1.17476476e-35, 1.78799641e-35, 2.78046720e-35, 4.39140622e-35, 6.89915883e-35, 1.08456398e-34, 1.72340852e-34, 2.76529889e-34, 4.47341414e-34, 7.19858182e-34, 1.15428925e-33, 1.86109869e-33, 3.02787711e-33, 4.99968788e-33, 8.41292126e-33, 1.48782986e-32, 2.81928746e-32, 5.81809245e-32, 1.19098362e-31, 2.23609340e-31, 4.31219441e-31, 9.09377071e-31, 2.13157327e-30, 5.18667520e-30, 1.27293168e-29, 3.26932839e-29, 8.74816036e-29, 2.31811202e-28, 6.21847530e-28, 1.75215848e-27, 5.30076177e-27, 1.66241054e-26, 5.02360630e-26, 1.45755206e-25, 4.25633786e-25, 1.21350043e-24, 3.15996087e-24, 7.49777016e-24, 1.63216285e-23, 3.45225687e-23, 7.33628099e-23, 1.56602795e-22, 3.31509646e-22, 6.77901960e-22, 1.33093704e-21, 2.47671423e-21, 4.36822472e-21, 7.30501018e-21, 1.16450550e-20, 1.78638063e-20, 2.60709438e-20, 3.54834135e-20, 4.46106487e-20, 5.24165238e-20, 5.95707126e-20, 6.71933283e-20, 7.62473807e-20, 8.75620764e-20, 1.01607433e-19, 1.20890024e-19, 1.49183271e-19, 1.93827895e-19, 2.69737524e-19, 4.04038653e-19, 6.64648342e-19, 1.22550521e-18, 2.58139627e-18, 6.30707613e-18, 1.79746450e-17, 5.95322037e-17, 2.25164621e-16, 9.92499171e-16, 5.14465327e-15, 3.01787479e-14, 1.55211562e-13, 7.10707186e-13, 2.92889900e-12, 1.10152924e-11, 3.79533676e-11, 1.20808007e-10])
- H2+(pressure)float644.502e-38 4.502e-38 ... 4.91e-20
- units :
- v/v
array([4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 6.48009717e-38, 4.72887596e-37, 4.73801629e-36, 1.23887304e-34, 4.97832988e-33, 3.19171929e-31, 3.07376875e-29, 2.13563147e-27, 1.09647217e-25, 4.20773047e-24, 1.23604260e-22, 2.78184517e-21, 4.91004692e-20])
- H3+(pressure)float644.502e-38 4.502e-38 ... 2.42e-15
- units :
- v/v
array([4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 4.50170000e-38, 6.98468161e-38, 2.77552966e-37, 9.48199378e-37, 3.09420977e-36, 1.02203266e-35, 3.43786422e-35, 1.17497722e-34, 3.77363714e-34, 1.12214004e-33, 3.01021532e-33, 7.28603174e-33, 1.60331412e-32, 3.23925342e-32, 6.08934338e-32, 1.03936121e-31, 1.55170071e-31, 1.99170124e-31, 2.27961993e-31, 2.44922527e-31, 2.59384861e-31, 2.77762272e-31, 3.04105537e-31, 3.46310399e-31, 4.13470952e-31, 5.27700636e-31, 7.39627866e-31, 1.18275494e-30, 2.20368181e-30, 4.88886011e-30, 1.33214179e-29, 4.60766812e-29, 2.08275915e-28, 1.25399725e-27, 9.85996310e-27, 1.00112582e-25, 1.36871346e-24, 2.58927396e-23, 6.52388099e-22, 1.31927132e-20, 2.17280673e-19, 2.92987417e-18, 3.28573365e-17, 3.06515314e-16, 2.42003680e-15])
- He(pressure)float640.1621 0.1621 ... 0.1625 0.163
- units :
- v/v
array([0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208016, 0.16208071, 0.16208072, 0.16208014, 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.16208 , 0.1620799 , 0.16207986, 0.1620808 , 0.16208123, 0.16208101, 0.16208026, 0.16207992, 0.16208316, 0.16208665, 0.16208888, 0.16209038, 0.16209205, 0.16211018, 0.16212839, 0.16214652, 0.16216425, 0.16218125, 0.16221971, 0.16225864, 0.16229578, 0.16233032, 0.16235901, 0.16238084, 0.16240129, 0.1624215 , 0.16244235, 0.16246186, 0.16246306, 0.162452 , 0.16241528, 0.16235583, 0.16227497, 0.16216587, 0.16209225, 0.16208646, 0.1621195 , 0.16224476, 0.16249357, 0.16298903])
- H2O(pressure)float640.001107 0.001106 ... 0.001591
- units :
- v/v
array([0.0011066 , 0.00110627, 0.00110615, 0.00110614, 0.00110621, 0.00110636, 0.00110655, 0.00110676, 0.00110698, 0.00110721, 0.00110738, 0.00110752, 0.00110767, 0.00110783, 0.001108 , 0.0011082 , 0.00110842, 0.00110863, 0.00110884, 0.00110905, 0.00110936, 0.00110971, 0.00111002, 0.00111029, 0.00111052, 0.00111167, 0.00111245, 0.00111268, 0.00111225, 0.00111161, 0.00111245, 0.00111328, 0.00111391, 0.0011143 , 0.00111516, 0.00111772, 0.00111782, 0.00111531, 0.00111492, 0.00111536, 0.00111747, 0.00111779, 0.00111625, 0.00111552, 0.00111686, 0.00111824, 0.00111794, 0.00111705, 0.00111751, 0.00111885, 0.00112084, 0.00112128, 0.00112051, 0.00112073, 0.00112456, 0.00112757, 0.00112841, 0.00112746, 0.00112782, 0.0011369 , 0.00114805, 0.00115703, 0.00116491, 0.00117354, 0.00122349, 0.00127572, 0.00132992, 0.00138529, 0.00144087, 0.00154128, 0.00164984, 0.00176242, 0.00187752, 0.0019764 , 0.00203319, 0.00208478, 0.002142 , 0.00221049, 0.00228784, 0.00232679, 0.00234517, 0.00223818, 0.00199768, 0.0016762 , 0.00166312, 0.00172987, 0.00165145, 0.00161002, 0.0015906 , 0.00158438, 0.00159066])
- CH4(pressure)float643.571e-11 6.89e-11 ... 0.001538
- units :
- v/v
array([3.57145162e-11, 6.89037815e-11, 1.18685480e-10, 1.91651855e-10, 2.94422816e-10, 4.35788866e-10, 6.29880583e-10, 8.99594027e-10, 1.27825882e-09, 1.80891238e-09, 2.54873258e-09, 3.55678782e-09, 4.90131874e-09, 6.68304646e-09, 9.04365997e-09, 1.22084159e-08, 1.64418833e-08, 2.20500739e-08, 2.94628711e-08, 3.92549179e-08, 5.21519488e-08, 6.92327934e-08, 9.19664587e-08, 1.22036876e-07, 1.61331953e-07, 2.10965899e-07, 2.70860909e-07, 3.38906469e-07, 4.10647759e-07, 4.98532978e-07, 6.15684415e-07, 7.50470160e-07, 8.75802494e-07, 9.70873902e-07, 1.05529188e-06, 1.13606365e-06, 1.20540743e-06, 1.26393129e-06, 1.31865026e-06, 1.36354082e-06, 1.37061779e-06, 1.33508095e-06, 1.28277048e-06, 1.25290752e-06, 1.24273823e-06, 1.22821368e-06, 1.23259483e-06, 1.29717478e-06, 1.42914312e-06, 1.64039169e-06, 1.90697052e-06, 2.21763512e-06, 2.57904600e-06, 3.01054831e-06, 3.55236510e-06, 4.28282971e-06, 5.32218325e-06, 6.81288036e-06, 8.93685575e-06, 1.18275361e-05, 1.58999061e-05, 2.19925612e-05, 3.15499490e-05, 4.70099420e-05, 6.70765315e-05, 9.73158293e-05, 1.41804655e-04, 2.06454041e-04, 2.99914498e-04, 3.76699469e-04, 4.67256511e-04, 5.78864001e-04, 7.17365257e-04, 8.51331620e-04, 8.97423613e-04, 9.34979565e-04, 9.86699866e-04, 1.06445243e-03, 1.17550490e-03, 1.30431123e-03, 1.52428871e-03, 1.79582176e-03, 2.17314555e-03, 2.58839359e-03, 2.31559692e-03, 1.94084303e-03, 1.75788845e-03, 1.60989396e-03, 1.51795179e-03, 1.49182083e-03, 1.53840238e-03])
- CO(pressure)float640.001459 0.001459 ... 0.001451
- units :
- v/v
array([0.00145938, 0.00145904, 0.00145891, 0.0014589 , 0.00145898, 0.00145913, 0.00145933, 0.00145955, 0.00145977, 0.00146001, 0.00146017, 0.00146029, 0.00146042, 0.00146056, 0.00146071, 0.00146085, 0.00146098, 0.00146112, 0.00146128, 0.00146144, 0.00146152, 0.00146155, 0.00146162, 0.00146174, 0.0014619 , 0.00146113, 0.00146077, 0.00146102, 0.00146203, 0.00146318, 0.00146261, 0.00146209, 0.00146181, 0.00146183, 0.00146136, 0.00145915, 0.00145953, 0.00146264, 0.0014634 , 0.00146326, 0.00146144, 0.00146148, 0.00146344, 0.00146465, 0.00146371, 0.00146272, 0.00146342, 0.00146474, 0.00146478, 0.00146387, 0.00146229, 0.00146231, 0.00146351, 0.0014634 , 0.00145955, 0.00145659, 0.00145585, 0.00145697, 0.00145682, 0.00144733, 0.00143588, 0.00142685, 0.00141906, 0.00141036, 0.00134707, 0.00128707, 0.00123028, 0.00117743, 0.00112925, 0.00097154, 0.00083626, 0.00073014, 0.00065181, 0.00056967, 0.00044896, 0.00036448, 0.00031699, 0.00030141, 0.00031549, 0.0003503 , 0.00043514, 0.00054867, 0.00071409, 0.000928 , 0.00113996, 0.00129026, 0.00136255, 0.0014024 , 0.00142518, 0.00143997, 0.00145117])
- NH3(pressure)float643.697e-11 4.896e-11 ... 0.0001434
- units :
- v/v
array([3.69676343e-11, 4.89600083e-11, 6.30976114e-11, 8.00641235e-11, 1.00381148e-10, 1.24732377e-10, 1.54106730e-10, 1.89851536e-10, 2.33600173e-10, 2.87147619e-10, 3.52547763e-10, 4.31798632e-10, 5.27248950e-10, 6.42149159e-10, 7.80651865e-10, 9.48474857e-10, 1.15172257e-09, 1.39709252e-09, 1.69322997e-09, 2.05071100e-09, 2.48203324e-09, 3.00356254e-09, 3.63517507e-09, 4.39841198e-09, 5.31695362e-09, 6.41256848e-09, 7.69852405e-09, 9.18268100e-09, 1.08650844e-08, 1.28591484e-08, 1.52869528e-08, 1.81149335e-08, 2.12373226e-08, 2.45848549e-08, 2.83288034e-08, 3.25873580e-08, 3.73172405e-08, 4.25665793e-08, 4.85104360e-08, 5.51761903e-08, 6.23634324e-08, 6.98959256e-08, 7.80189042e-08, 8.74302175e-08, 9.84064378e-08, 1.10661691e-07, 1.24834589e-07, 1.42435212e-07, 1.64409526e-07, 1.91777445e-07, 2.24456267e-07, 2.62566098e-07, 3.06994444e-07, 3.59347774e-07, 4.22332824e-07, 4.98848012e-07, 5.93182104e-07, 7.10084356e-07, 8.55543323e-07, 1.03647955e-06, 1.26147463e-06, 1.54491542e-06, 1.90848672e-06, 2.38073792e-06, 2.99005480e-06, 3.77073072e-06, 4.75967628e-06, 6.00372242e-06, 7.56216036e-06, 9.48133898e-06, 1.18502410e-05, 1.47489080e-05, 1.82489583e-05, 2.23476562e-05, 2.69815324e-05, 3.22443707e-05, 3.80792944e-05, 4.43652420e-05, 5.09137698e-05, 5.74532424e-05, 6.38740116e-05, 6.99600636e-05, 7.53398898e-05, 7.96413334e-05, 8.28572830e-05, 8.75267759e-05, 9.38342536e-05, 1.02035861e-04, 1.12530167e-04, 1.25938666e-04, 1.43395692e-04])
- N2(pressure)float640.0002165 0.0002165 ... 0.0002038
- units :
- v/v
array([0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021645, 0.00021644, 0.00021644, 0.00021644, 0.00021644, 0.00021644, 0.00021644, 0.00021644, 0.00021644, 0.00021644, 0.00021644, 0.00021643, 0.00021643, 0.00021643, 0.00021642, 0.00021642, 0.00021642, 0.00021641, 0.0002164 , 0.0002164 , 0.0002164 , 0.00021639, 0.00021638, 0.00021637, 0.00021636, 0.00021636, 0.00021634, 0.00021631, 0.00021629, 0.00021627, 0.00021625, 0.0002162 , 0.00021613, 0.00021608, 0.00021603, 0.00021599, 0.00021585, 0.00021568, 0.00021552, 0.00021537, 0.0002152 , 0.00021468, 0.00021415, 0.00021363, 0.00021312, 0.00021261, 0.00021112, 0.00020962, 0.00020819, 0.00020688, 0.00020481, 0.00020088, 0.00019741, 0.00019463, 0.00019274, 0.00019177, 0.00019129, 0.00019177, 0.0001925 , 0.00019367, 0.00019516, 0.00019692, 0.00019848, 0.00019976, 0.00020087, 0.00020183, 0.00020275, 0.00020379])
- PH3(pressure)float641.849e-15 1.902e-15 ... 1.162e-06
- units :
- v/v
array([1.84868779e-15, 1.90204827e-15, 2.14578036e-15, 2.55110235e-15, 3.15838024e-15, 4.03018193e-15, 5.24291455e-15, 6.88718086e-15, 9.08472631e-15, 1.20233185e-14, 1.59501764e-14, 2.13068665e-14, 2.87506195e-14, 3.91238796e-14, 5.35629969e-14, 7.34706639e-14, 1.00965655e-13, 1.39219940e-13, 1.92533204e-13, 2.66875691e-13, 3.70668880e-13, 5.15072250e-13, 7.15444584e-13, 9.94706082e-13, 1.38728421e-12, 1.94713776e-12, 2.77598045e-12, 4.04612874e-12, 6.06164996e-12, 9.06174615e-12, 1.32717438e-11, 1.96395515e-11, 3.00886278e-11, 4.80259258e-11, 7.77530249e-11, 1.26279699e-10, 2.08783424e-10, 3.50793688e-10, 5.85633174e-10, 9.81501415e-10, 1.67399176e-09, 2.93884252e-09, 5.23949443e-09, 9.01573208e-09, 1.51522516e-08, 2.55932903e-08, 4.30756125e-08, 6.50902926e-08, 8.13752097e-08, 1.02679298e-07, 1.30380807e-07, 1.68226424e-07, 2.14547893e-07, 2.40959274e-07, 2.66345567e-07, 2.95326000e-07, 3.31795299e-07, 3.77396643e-07, 4.31209104e-07, 4.78979687e-07, 5.27367941e-07, 5.87132371e-07, 6.61916055e-07, 7.54591288e-07, 8.12295752e-07, 8.78294376e-07, 9.50787393e-07, 1.02914386e-06, 1.11352480e-06, 1.15652397e-06, 1.19657578e-06, 1.23681991e-06, 1.27711858e-06, 1.31004644e-06, 1.32083944e-06, 1.32321881e-06, 1.32378459e-06, 1.32371743e-06, 1.32067041e-06, 1.30411146e-06, 1.29080141e-06, 1.26411062e-06, 1.22745368e-06, 1.17503044e-06, 1.09920607e-06, 1.03968744e-06, 9.99183778e-07, 9.84514851e-07, 1.00211885e-06, 1.05994455e-06, 1.16211896e-06])
- H2S(pressure)float648.59e-05 8.573e-05 ... 9.658e-05
- units :
- v/v
array([8.59018107e-05, 8.57344303e-05, 8.55665764e-05, 8.54267864e-05, 8.53396948e-05, 8.53462577e-05, 8.54498176e-05, 8.55853595e-05, 8.57454431e-05, 8.59297171e-05, 8.60065576e-05, 8.60059476e-05, 8.60054812e-05, 8.60051915e-05, 8.60051086e-05, 8.57707661e-05, 8.54407932e-05, 8.51587512e-05, 8.49255442e-05, 8.47417145e-05, 8.45985966e-05, 8.44995613e-05, 8.44517679e-05, 8.44568026e-05, 8.45185658e-05, 8.47263524e-05, 8.49884230e-05, 8.53095416e-05, 8.57036239e-05, 8.60076295e-05, 8.60068390e-05, 8.60062260e-05, 8.60057131e-05, 8.60053358e-05, 8.59608700e-05, 8.58096181e-05, 8.58081651e-05, 8.59650993e-05, 8.60013827e-05, 8.59986804e-05, 8.59963203e-05, 8.59941665e-05, 8.59924089e-05, 8.59825561e-05, 8.59717089e-05, 8.59624878e-05, 8.59560835e-05, 8.59456149e-05, 8.59265220e-05, 8.59159758e-05, 8.59068882e-05, 8.59013333e-05, 8.58938201e-05, 8.58694138e-05, 8.58513768e-05, 8.58393658e-05, 8.58357376e-05, 8.58396504e-05, 8.58246321e-05, 8.58121124e-05, 8.58064631e-05, 8.58121055e-05, 8.58287577e-05, 8.58545205e-05, 8.58777628e-05, 8.59031350e-05, 8.59290487e-05, 8.59547807e-05, 8.59800981e-05, 8.60082499e-05, 8.60357987e-05, 8.60621892e-05, 8.60870525e-05, 8.61075276e-05, 8.61182144e-05, 8.61224175e-05, 8.61268379e-05, 8.61329240e-05, 8.61356425e-05, 8.61331603e-05, 8.61993085e-05, 8.67142801e-05, 8.80745809e-05, 8.57822960e-05, 7.98177871e-05, 7.71524951e-05, 7.80130157e-05, 7.98125564e-05, 8.30209869e-05, 8.82193549e-05, 9.65806857e-05])
- TiO(pressure)float641.194e-28 5.244e-29 ... 3.811e-07
- units :
- v/v
array([1.19363013e-28, 5.24372322e-29, 3.30779978e-29, 2.56393309e-29, 2.33011518e-29, 2.38446777e-29, 2.63235691e-29, 3.01906704e-29, 3.51944757e-29, 4.15659640e-29, 4.96509297e-29, 6.10398640e-29, 7.80928824e-29, 1.03305696e-28, 1.39971439e-28, 1.91094343e-28, 2.62828427e-28, 3.66353238e-28, 5.16620911e-28, 7.35174656e-28, 1.05506684e-27, 1.51738046e-27, 2.17840003e-27, 3.13851721e-27, 4.57695221e-27, 6.87339903e-27, 1.09612855e-26, 1.90277004e-26, 3.67034799e-26, 7.02805397e-26, 1.25616862e-25, 2.33950349e-25, 4.99908855e-25, 1.25649305e-24, 3.34969286e-24, 9.11516601e-24, 2.63260820e-23, 8.00915300e-23, 2.42300229e-22, 7.48736170e-22, 2.50252492e-21, 9.31594453e-21, 3.65682519e-20, 1.34977727e-19, 4.67981772e-19, 1.64042397e-18, 5.53602593e-18, 1.61598638e-17, 4.04991934e-17, 8.90404683e-17, 1.87591309e-16, 3.98244100e-16, 8.50382815e-16, 1.77908714e-15, 3.52941159e-15, 6.58651759e-15, 1.13394064e-14, 1.80066578e-14, 2.63163504e-14, 3.56286490e-14, 4.54876647e-14, 5.39035710e-14, 5.75089774e-14, 5.44130806e-14, 4.56471654e-14, 3.64176614e-14, 2.87296418e-14, 2.28805375e-14, 1.85680071e-14, 1.51420753e-14, 1.28137421e-14, 1.14579422e-14, 1.10704116e-14, 1.19760332e-14, 1.48045027e-14, 2.10130457e-14, 3.51100330e-14, 7.08059260e-14, 1.76114996e-13, 5.55962915e-13, 2.18652689e-12, 9.78629345e-12, 5.53977490e-11, 4.01178482e-10, 3.10142566e-09, 3.25746635e-08, 3.08308759e-07, 4.51590489e-07, 4.35573005e-07, 4.12525085e-07, 3.81122368e-07])
- VO(pressure)float641.291e-24 6.074e-25 ... 6.752e-09
- units :
- v/v
array([1.29123157e-24, 6.07426379e-25, 3.93331224e-25, 3.05520906e-25, 2.73121288e-25, 2.71138077e-25, 2.87813685e-25, 3.15987890e-25, 3.51946161e-25, 3.96535045e-25, 4.51245819e-25, 5.26704603e-25, 6.36805552e-25, 7.92981219e-25, 1.00856533e-24, 1.29138420e-24, 1.66436125e-24, 2.17051740e-24, 2.85977826e-24, 3.79826850e-24, 5.08261952e-24, 6.81413316e-24, 9.12115783e-24, 1.22475657e-23, 1.66224739e-23, 2.31551592e-23, 3.40107448e-23, 5.38358494e-23, 9.35231305e-23, 1.61406441e-22, 2.62162609e-22, 4.41559156e-22, 8.39634712e-22, 1.84253602e-21, 4.25942475e-21, 1.00291297e-20, 2.48799532e-20, 6.45955527e-20, 1.66923903e-19, 4.39551880e-19, 1.24075966e-18, 3.85093200e-18, 1.25215276e-17, 3.85686817e-17, 1.12447316e-16, 3.31028663e-16, 9.42156265e-16, 2.35908627e-15, 5.16444260e-15, 1.00728376e-14, 1.89222719e-14, 3.57783088e-14, 6.79886537e-14, 1.26922691e-13, 2.26197690e-13, 3.81888705e-13, 6.00239920e-13, 8.78235523e-13, 1.19452200e-12, 1.52053460e-12, 1.83828404e-12, 2.08014824e-12, 2.14495382e-12, 1.98936645e-12, 1.66831594e-12, 1.33838844e-12, 1.06306878e-12, 8.51373453e-13, 6.93086047e-13, 5.69262939e-13, 4.83152689e-13, 4.30385736e-13, 4.10336616e-13, 4.31462523e-13, 5.08529523e-13, 6.76910939e-13, 1.04110303e-12, 1.89215867e-12, 4.14342745e-12, 1.11087287e-11, 3.60810193e-11, 1.42321431e-10, 7.05812138e-10, 7.15089944e-09, 2.30203481e-08, 2.13843941e-08, 1.80340327e-08, 1.43635533e-08, 1.11671893e-08, 8.65764143e-09, 6.75173919e-09])
- Fe(pressure)float643.182e-14 1.784e-14 ... 0.0001611
- units :
- v/v
array([3.18180026e-14, 1.78380818e-14, 1.23520589e-14, 9.64612536e-15, 8.26612665e-15, 7.59166366e-15, 7.28782299e-15, 7.15373565e-15, 7.08918744e-15, 7.07887494e-15, 7.11541485e-15, 7.27321593e-15, 7.60949863e-15, 8.11814089e-15, 8.78269639e-15, 9.54388350e-15, 1.04160078e-14, 1.14568107e-14, 1.26873084e-14, 1.41246582e-14, 1.58032133e-14, 1.77035791e-14, 1.98115956e-14, 2.22162928e-14, 2.50893255e-14, 2.88335387e-14, 3.43139861e-14, 4.28985635e-14, 5.70193836e-14, 7.54471388e-14, 9.59201855e-14, 1.24906793e-13, 1.76207098e-13, 2.73221917e-13, 4.38529332e-13, 7.12802398e-13, 1.19837755e-12, 2.07460214e-12, 3.57234958e-12, 6.22607871e-12, 1.13640409e-11, 2.20651993e-11, 4.41502648e-11, 8.50954407e-11, 1.58126966e-10, 2.95708656e-10, 5.40638186e-10, 9.08452942e-10, 1.39758805e-09, 1.99417239e-09, 2.77689159e-09, 3.88155342e-09, 5.44190256e-09, 7.54617290e-09, 1.01617602e-08, 1.32045039e-08, 1.63632444e-08, 1.93388175e-08, 2.18003297e-08, 2.35919780e-08, 2.46972959e-08, 2.47419708e-08, 2.33154355e-08, 2.05017331e-08, 1.70682932e-08, 1.38036065e-08, 1.10900822e-08, 8.95580385e-09, 7.30673389e-09, 6.05146637e-09, 5.12186621e-09, 4.47155162e-09, 4.07777003e-09, 3.94946941e-09, 4.09136456e-09, 4.58539012e-09, 5.65298098e-09, 7.78574482e-09, 1.21262761e-08, 2.14335968e-08, 4.28166324e-08, 9.52419525e-08, 2.34504600e-07, 6.48056964e-07, 1.98218124e-06, 6.34265919e-06, 3.02685763e-05, 1.64585606e-04, 1.63371503e-04, 1.62166143e-04, 1.61114485e-04])
- FeH(pressure)float642.089e-21 1.283e-21 ... 2.549e-05
- units :
- v/v
array([2.08884301e-21, 1.28304284e-21, 9.89822354e-22, 8.69425348e-22, 8.44185878e-22, 8.83303007e-22, 9.69447920e-22, 1.08988232e-21, 1.23791279e-21, 1.41764085e-21, 1.63534160e-21, 1.92121135e-21, 2.31449131e-21, 2.84764827e-21, 3.55692435e-21, 4.46415453e-21, 5.62900276e-21, 7.15783988e-21, 9.16879532e-21, 1.18121874e-20, 1.52997057e-20, 1.98440156e-20, 2.57087926e-20, 3.33809668e-20, 4.36744055e-20, 5.82309904e-20, 8.06235800e-20, 1.17729456e-19, 1.83675485e-19, 2.85228573e-19, 4.24443257e-19, 6.48196696e-19, 1.07944119e-18, 1.99115247e-18, 3.81264986e-18, 7.40088036e-18, 1.49002476e-17, 3.09643122e-17, 6.40557423e-17, 1.34288490e-16, 2.95977011e-16, 6.97562336e-16, 1.69844615e-15, 3.97670175e-15, 8.95570770e-15, 2.03081910e-14, 4.49346690e-14, 9.07551321e-14, 1.66699060e-13, 2.82110931e-13, 4.64929904e-13, 7.69396093e-13, 1.27762744e-12, 2.09824043e-12, 3.33751328e-12, 5.10628603e-12, 7.41865406e-12, 1.02353721e-11, 1.34191615e-11, 1.68290876e-11, 2.03543254e-11, 2.34637928e-11, 2.53000009e-11, 2.52938257e-11, 2.38204626e-11, 2.17334749e-11, 1.96872316e-11, 1.79338337e-11, 1.65204044e-11, 1.54692609e-11, 1.48325799e-11, 1.47118530e-11, 1.53037652e-11, 1.70016427e-11, 2.03315845e-11, 2.65094329e-11, 3.83637367e-11, 6.26750198e-11, 1.17174091e-10, 2.51930035e-10, 6.19616913e-10, 1.71810508e-09, 5.34857968e-09, 1.89855164e-08, 7.56459831e-08, 3.12569037e-07, 1.91119093e-06, 1.32088617e-05, 1.65515030e-05, 2.05933912e-05, 2.54912338e-05])
- CrH(pressure)float641.34e-20 8.305e-21 ... 3.329e-07
- units :
- v/v
array([1.34027308e-20, 8.30516882e-21, 6.42870321e-21, 5.64823582e-21, 5.47261037e-21, 5.70386676e-21, 6.22867679e-21, 6.96327410e-21, 7.86285402e-21, 8.95009379e-21, 1.02607101e-20, 1.19748127e-20, 1.43224082e-20, 1.74862942e-20, 2.16661849e-20, 2.69710007e-20, 3.37281355e-20, 4.25264245e-20, 5.40044420e-20, 6.89649622e-20, 8.85331522e-20, 1.13805411e-19, 1.46129467e-19, 1.88042290e-19, 2.43784658e-19, 3.21927845e-19, 4.41065901e-19, 6.36526796e-19, 9.79925044e-19, 1.50193619e-18, 2.20893755e-18, 3.33212271e-18, 5.47026486e-18, 9.92428169e-18, 1.86739924e-17, 3.56098729e-17, 7.03713757e-17, 1.43438545e-16, 2.45147305e-16, 4.09398937e-16, 7.09330987e-16, 1.29106266e-15, 2.40681263e-15, 5.47657340e-15, 1.35842499e-14, 3.39519819e-14, 8.25892572e-14, 1.80131022e-13, 3.47490429e-13, 6.14219994e-13, 1.05537974e-12, 1.82141408e-12, 3.14572464e-12, 5.29314625e-12, 8.61546643e-12, 1.34676446e-11, 1.99505242e-11, 2.80084043e-11, 3.70686882e-11, 4.67923692e-11, 5.69250011e-11, 6.59446308e-11, 7.13651876e-11, 7.15062605e-11, 6.74146756e-11, 6.15387773e-11, 5.57649018e-11, 5.08220295e-11, 4.68483072e-11, 4.39115048e-11, 4.21653909e-11, 4.19097859e-11, 4.37266313e-11, 4.87840443e-11, 5.85690581e-11, 7.64636136e-11, 1.10836501e-10, 1.81442209e-10, 3.39447354e-10, 7.24668772e-10, 1.77568274e-09, 5.16578643e-09, 2.37324008e-08, 6.92979907e-08, 8.98637470e-08, 1.14849165e-07, 1.44957204e-07, 1.80809529e-07, 2.23367929e-07, 2.73601535e-07, 3.32920226e-07])
- Na(pressure)float641.094e-05 1.047e-05 ... 1.08e-05
- units :
- v/v
array([1.09432271e-05, 1.04727327e-05, 9.96162531e-06, 9.52789435e-06, 9.24317311e-06, 9.14318262e-06, 9.23761953e-06, 9.52526533e-06, 1.00094177e-05, 1.07232348e-05, 1.10674552e-05, 1.10663504e-05, 1.10650994e-05, 1.10637071e-05, 1.10621869e-05, 1.05757188e-05, 9.92516720e-06, 9.39863356e-06, 8.98267003e-06, 8.66640330e-06, 8.10815820e-06, 7.49440203e-06, 7.09294672e-06, 6.87700893e-06, 6.83959376e-06, 6.99821818e-06, 7.42220070e-06, 8.22771607e-06, 9.62283753e-06, 1.10299120e-05, 1.10281096e-05, 1.10259977e-05, 1.10235271e-05, 1.10206183e-05, 1.09196395e-05, 1.05894324e-05, 1.05834796e-05, 1.09200881e-05, 1.10098701e-05, 1.10118324e-05, 1.10128855e-05, 1.10129724e-05, 1.10118511e-05, 1.10252954e-05, 1.10430185e-05, 1.10577471e-05, 1.10678280e-05, 1.10821652e-05, 1.11117904e-05, 1.11332543e-05, 1.11506446e-05, 1.11638926e-05, 1.11768196e-05, 1.12058056e-05, 1.12320902e-05, 1.12527398e-05, 1.12664925e-05, 1.12737621e-05, 1.12803164e-05, 1.12850337e-05, 1.12861487e-05, 1.12827715e-05, 1.12733466e-05, 1.12571895e-05, 1.12329911e-05, 1.12060147e-05, 1.11784193e-05, 1.11512362e-05, 1.11248411e-05, 1.10929783e-05, 1.10622100e-05, 1.10335895e-05, 1.10078477e-05, 1.09819215e-05, 1.09528366e-05, 1.09349747e-05, 1.09250828e-05, 1.09241999e-05, 1.09351595e-05, 1.09689123e-05, 1.10183492e-05, 1.10766187e-05, 1.11328116e-05, 1.11672611e-05, 1.11485777e-05, 1.10986894e-05, 1.10311383e-05, 1.09496126e-05, 1.08688600e-05, 1.08065672e-05, 1.08021181e-05])
- K(pressure)float644.198e-07 4.095e-07 ... 7.844e-07
- units :
- v/v
array([4.19760063e-07, 4.09511287e-07, 3.99385595e-07, 3.91070991e-07, 3.85890058e-07, 3.83820304e-07, 3.84985514e-07, 3.90881394e-07, 4.01441881e-07, 4.17152422e-07, 4.25419471e-07, 4.26649806e-07, 4.27998338e-07, 4.29444071e-07, 4.30956522e-07, 4.25109330e-07, 4.16390713e-07, 4.09294956e-07, 4.03780212e-07, 3.99805696e-07, 3.88628851e-07, 3.74660470e-07, 3.65351131e-07, 3.60463645e-07, 3.60065667e-07, 3.61634056e-07, 3.70825544e-07, 3.90494767e-07, 4.24657070e-07, 4.58575383e-07, 4.60892332e-07, 4.63326120e-07, 4.66254382e-07, 4.69773895e-07, 4.72001748e-07, 4.70634409e-07, 4.74620525e-07, 4.84370819e-07, 4.91018971e-07, 4.96227680e-07, 5.01564798e-07, 5.07264325e-07, 5.13076149e-07, 5.21430317e-07, 5.30323336e-07, 5.38773246e-07, 5.46262041e-07, 5.54018497e-07, 5.64142380e-07, 5.72160150e-07, 5.79295744e-07, 5.85668868e-07, 5.91938551e-07, 6.01050325e-07, 6.09580520e-07, 6.16816656e-07, 6.22339972e-07, 6.26192959e-07, 6.29092172e-07, 6.31294589e-07, 6.32620718e-07, 6.32786932e-07, 6.31328718e-07, 6.28023750e-07, 6.22326300e-07, 6.15919872e-07, 6.09407350e-07, 6.03090923e-07, 5.97092532e-07, 5.89219214e-07, 5.81843738e-07, 5.75364821e-07, 5.70090871e-07, 5.65583579e-07, 5.61024572e-07, 5.59157441e-07, 5.60098662e-07, 5.64371247e-07, 5.72597798e-07, 5.86315044e-07, 6.05660106e-07, 6.28394619e-07, 6.52570907e-07, 6.73825645e-07, 6.84413364e-07, 6.88714955e-07, 6.91562799e-07, 6.95713956e-07, 7.07634205e-07, 7.33978002e-07, 7.84440904e-07])
- Rb(pressure)float647.307e-10 7.112e-10 ... 1.583e-09
- units :
- v/v
array([7.30701085e-10, 7.11154725e-10, 6.92072109e-10, 6.76520006e-10, 6.66924120e-10, 6.63410227e-10, 6.66194876e-10, 6.77610577e-10, 6.97542994e-10, 7.26929020e-10, 7.42522734e-10, 7.45130649e-10, 7.47975836e-10, 7.51015496e-10, 7.54187618e-10, 7.43146632e-10, 7.26580336e-10, 7.13159449e-10, 7.02789764e-10, 6.95382585e-10, 6.74853277e-10, 6.49345406e-10, 6.32518162e-10, 6.23907396e-10, 6.23638844e-10, 6.27852817e-10, 6.46068425e-10, 6.83614918e-10, 7.48191468e-10, 8.12405572e-10, 8.17415035e-10, 8.22657937e-10, 8.28869298e-10, 8.36237883e-10, 8.41042479e-10, 8.38775934e-10, 8.47034283e-10, 8.66668553e-10, 8.80361658e-10, 8.91229741e-10, 9.02460794e-10, 9.14355614e-10, 9.26395444e-10, 9.43755727e-10, 9.62371427e-10, 9.80117621e-10, 9.95821263e-10, 1.01210871e-09, 1.03346062e-09, 1.05045894e-09, 1.06568556e-09, 1.07926317e-09, 1.09262187e-09, 1.11219156e-09, 1.13065301e-09, 1.14635093e-09, 1.15832840e-09, 1.16667353e-09, 1.17308579e-09, 1.17826554e-09, 1.18162556e-09, 1.18244339e-09, 1.17974695e-09, 1.17310147e-09, 1.16208057e-09, 1.14953604e-09, 1.13675276e-09, 1.12436084e-09, 1.11260387e-09, 1.09824246e-09, 1.08489062e-09, 1.07322411e-09, 1.06380573e-09, 1.05603885e-09, 1.04893068e-09, 1.04732673e-09, 1.05100911e-09, 1.06092004e-09, 1.07840198e-09, 1.10741214e-09, 1.14753233e-09, 1.19498283e-09, 1.24533779e-09, 1.28983960e-09, 1.31279291e-09, 1.32407630e-09, 1.33537242e-09, 1.35492786e-09, 1.39600077e-09, 1.47129377e-09, 1.58276792e-09])
- Cs(pressure)float646.688e-12 6.344e-12 ... 7.608e-11
- units :
- v/v
array([6.68760759e-12, 6.34378579e-12, 6.06644812e-12, 5.86643321e-12, 5.76387462e-12, 5.76546336e-12, 5.86974161e-12, 6.07708961e-12, 6.38674601e-12, 6.81487200e-12, 7.07583423e-12, 7.18067404e-12, 7.29647641e-12, 7.42200132e-12, 7.55516990e-12, 7.44906655e-12, 7.24926569e-12, 7.10330851e-12, 7.00902473e-12, 6.96471364e-12, 6.76745598e-12, 6.51703006e-12, 6.38400013e-12, 6.36478961e-12, 6.46644145e-12, 6.69616000e-12, 7.13754626e-12, 7.88919046e-12, 9.11284686e-12, 1.03778951e-11, 1.06551159e-11, 1.09525328e-11, 1.13094034e-11, 1.17404628e-11, 1.21346849e-11, 1.23641180e-11, 1.28891130e-11, 1.37598886e-11, 1.45094086e-11, 1.52109800e-11, 1.59754847e-11, 1.68251539e-11, 1.77324385e-11, 1.88875815e-11, 2.01327642e-11, 2.14034932e-11, 2.26209209e-11, 2.38917012e-11, 2.54538602e-11, 2.67692267e-11, 2.80137091e-11, 2.91896538e-11, 3.03973316e-11, 3.21053340e-11, 3.37708918e-11, 3.52515882e-11, 3.64375012e-11, 3.73157498e-11, 3.80648412e-11, 3.87379835e-11, 3.92472821e-11, 3.95006166e-11, 3.94101008e-11, 3.89463380e-11, 3.81828068e-11, 3.72885805e-11, 3.63817062e-11, 3.55165752e-11, 3.47115288e-11, 3.38410425e-11, 3.30628477e-11, 3.24140369e-11, 3.19303993e-11, 3.16144220e-11, 3.14690936e-11, 3.17208569e-11, 3.23361028e-11, 3.33882020e-11, 3.50131945e-11, 3.76412372e-11, 4.12857115e-11, 4.60736171e-11, 5.17339995e-11, 5.75122892e-11, 6.14656090e-11, 6.41902601e-11, 6.66414009e-11, 6.91419930e-11, 7.17965476e-11, 7.45566522e-11, 7.60804551e-11])
- CO2(pressure)float648.698e-06 8.966e-06 ... 3.76e-07
- units :
- v/v
array([8.69837845e-06, 8.96596991e-06, 9.07043977e-06, 9.07886258e-06, 9.01280517e-06, 8.89247649e-06, 8.73941058e-06, 8.57203262e-06, 8.40077172e-06, 8.22737473e-06, 8.05429685e-06, 7.87417305e-06, 7.68243799e-06, 7.48260213e-06, 7.27906417e-06, 7.07835375e-06, 6.88058960e-06, 6.68378724e-06, 6.48876222e-06, 6.29650247e-06, 6.10742093e-06, 5.92343140e-06, 5.74545960e-06, 5.57177958e-06, 5.39996327e-06, 5.22641129e-06, 5.04262146e-06, 4.84380191e-06, 4.62729916e-06, 4.42310519e-06, 4.24663260e-06, 4.06881629e-06, 3.87161313e-06, 3.65402972e-06, 3.43876436e-06, 3.23369119e-06, 3.03067297e-06, 2.83190803e-06, 2.65122575e-06, 2.48042427e-06, 2.31241093e-06, 2.14381259e-06, 1.98174243e-06, 1.83997381e-06, 1.71521435e-06, 1.59805306e-06, 1.49112839e-06, 1.40149730e-06, 1.32851030e-06, 1.26720933e-06, 1.21128110e-06, 1.15710555e-06, 1.10498862e-06, 1.05712454e-06, 1.01435126e-06, 9.75906207e-07, 9.42060925e-07, 9.12490278e-07, 8.87831938e-07, 8.67842275e-07, 8.50743636e-07, 8.36534453e-07, 8.26301480e-07, 8.20475708e-07, 8.13729430e-07, 8.09221943e-07, 8.05340551e-07, 8.01478312e-07, 7.97546383e-07, 7.31389018e-07, 6.70398966e-07, 6.20538346e-07, 5.83715565e-07, 5.28708841e-07, 4.19893832e-07, 3.40476986e-07, 2.94243987e-07, 2.76921307e-07, 2.85148077e-07, 3.03478367e-07, 3.54941866e-07, 3.96333785e-07, 4.23749565e-07, 4.21869731e-07, 4.67215529e-07, 5.04463159e-07, 4.70324755e-07, 4.39988108e-07, 4.14362089e-07, 3.93039788e-07, 3.75968303e-07])
- HCN(pressure)float645.674e-14 7.279e-14 ... 1.084e-05
- units :
- v/v
array([5.67389785e-14, 7.27874146e-14, 9.26695269e-14, 1.17474069e-13, 1.48422066e-13, 1.87055647e-13, 2.35367302e-13, 2.95919715e-13, 3.71923223e-13, 4.67319513e-13, 5.86551932e-13, 7.35317008e-13, 9.20922213e-13, 1.15244256e-12, 1.44132975e-12, 1.80224176e-12, 2.25309068e-12, 2.81582935e-12, 3.51815129e-12, 4.39470659e-12, 5.48764469e-12, 6.85145199e-12, 8.55511650e-12, 1.06821866e-11, 1.33351021e-11, 1.66107575e-11, 2.06736953e-11, 2.57017132e-11, 3.19070668e-11, 3.96011884e-11, 4.90219137e-11, 6.06183712e-11, 7.47045682e-11, 9.16983087e-11, 1.12290233e-10, 1.37050261e-10, 1.67676746e-10, 2.05734434e-10, 2.50958407e-10, 3.05370933e-10, 3.69724759e-10, 4.47532193e-10, 5.42512249e-10, 6.57078215e-10, 7.94038551e-10, 9.59159848e-10, 1.16311974e-09, 1.41774440e-09, 1.73156040e-09, 2.12039671e-09, 2.59725311e-09, 3.18866065e-09, 3.92139629e-09, 4.81614350e-09, 5.88983002e-09, 7.22671250e-09, 8.92119792e-09, 1.10737129e-08, 1.37551713e-08, 1.68796313e-08, 2.06839216e-08, 2.54992015e-08, 3.15986643e-08, 3.92539027e-08, 4.53541030e-08, 5.24968899e-08, 6.08250921e-08, 7.06003227e-08, 8.21985509e-08, 8.31523342e-08, 8.39920749e-08, 8.61077805e-08, 9.03271141e-08, 9.34148372e-08, 8.84309376e-08, 8.62669672e-08, 8.96714278e-08, 1.01086276e-07, 1.24604149e-07, 1.65219115e-07, 2.46572315e-07, 3.93902456e-07, 6.93395178e-07, 1.29543459e-06, 1.93230547e-06, 2.55941027e-06, 3.49659676e-06, 4.62109255e-06, 6.05704715e-06, 7.99796551e-06, 1.08413672e-05])
- C2H2(pressure)float647.779e-22 1.296e-21 ... 8.129e-06
- units :
- v/v
array([7.77890904e-22, 1.29611942e-21, 2.11084693e-21, 3.39330681e-21, 5.40043957e-21, 8.53061835e-21, 1.34106685e-20, 2.10317703e-20, 3.29500669e-20, 5.15799606e-20, 8.06538339e-20, 1.25850504e-19, 1.95869343e-19, 3.04186709e-19, 4.71674037e-19, 7.30978625e-19, 1.13226812e-18, 1.75236631e-18, 2.71007142e-18, 4.18878275e-18, 6.46860412e-18, 9.98607454e-18, 1.54200623e-17, 2.38087045e-17, 3.67371865e-17, 5.64160510e-17, 8.64079405e-17, 1.31869391e-16, 2.00336893e-16, 3.04562969e-16, 4.62272516e-16, 6.99797982e-16, 1.05053667e-15, 1.56161102e-15, 2.30874885e-15, 3.39008978e-15, 4.99863201e-15, 7.40809951e-15, 1.08904766e-14, 1.59390741e-14, 2.30805714e-14, 3.33741897e-14, 4.83778924e-14, 7.01796939e-14, 1.01505850e-13, 1.46689505e-13, 2.13682899e-13, 3.14902453e-13, 4.66733344e-13, 6.95947543e-13, 1.03861662e-12, 1.55703201e-12, 2.34208475e-12, 3.51445391e-12, 5.23133121e-12, 7.84126321e-12, 1.19018441e-11, 1.82720974e-11, 2.80963193e-11, 4.22020070e-11, 6.32400513e-11, 9.59536799e-11, 1.47193876e-10, 2.27090546e-10, 3.04011531e-10, 4.08584432e-10, 5.50253983e-10, 7.43620449e-10, 1.01094094e-09, 1.04349413e-09, 1.07383501e-09, 1.13745735e-09, 1.26004110e-09, 1.36047247e-09, 1.24083432e-09, 1.19766106e-09, 1.30649838e-09, 1.66659604e-09, 2.52434369e-09, 4.39233970e-09, 9.60273895e-09, 2.38483951e-08, 7.11619761e-08, 2.36327572e-07, 4.96032050e-07, 8.16622832e-07, 1.41185441e-06, 2.25678756e-06, 3.48187708e-06, 5.31470203e-06, 8.12936301e-06])
- C2H4(pressure)float641.263e-22 3.107e-22 ... 2.466e-06
- units :
- v/v
array([1.26255185e-22, 3.10732314e-22, 6.79965663e-22, 1.39164226e-21, 2.70472281e-21, 5.05795774e-21, 9.22843670e-21, 1.66302557e-20, 2.98108013e-20, 5.32121802e-20, 9.45503013e-20, 1.66330838e-19, 2.88802221e-19, 4.95980111e-19, 8.45111366e-19, 1.43633112e-18, 2.43516348e-18, 4.11057801e-18, 6.91238925e-18, 1.15894924e-17, 1.93704922e-17, 3.23467484e-17, 5.40548126e-17, 9.02386898e-17, 1.50067671e-16, 2.46421395e-16, 3.97259387e-16, 6.24084893e-16, 9.49337928e-16, 1.44729024e-15, 2.24011859e-15, 3.42056773e-15, 4.99406862e-15, 6.91563819e-15, 9.37612283e-15, 1.25529590e-14, 1.66188313e-14, 2.18212292e-14, 2.84000072e-14, 3.65724983e-14, 4.56160005e-14, 5.52206053e-14, 6.60928309e-14, 8.03534506e-14, 9.89383872e-14, 1.21359465e-13, 1.51637725e-13, 1.99226530e-13, 2.73923430e-13, 3.92463210e-13, 5.69234480e-13, 8.27904353e-13, 1.20633780e-12, 1.76133917e-12, 2.58625857e-12, 3.88845290e-12, 6.05332669e-12, 9.74554486e-12, 1.60610269e-11, 2.63536186e-11, 4.38184264e-11, 7.53113682e-11, 1.34682905e-10, 2.50249114e-10, 4.13943213e-10, 6.96834889e-10, 1.17910665e-09, 1.99727093e-09, 3.38702072e-09, 4.32996965e-09, 5.46264713e-09, 6.99115871e-09, 9.16759640e-09, 1.13903982e-08, 1.15815153e-08, 1.20056055e-08, 1.34477731e-08, 1.67179505e-08, 2.32836283e-08, 3.50635991e-08, 6.26017442e-08, 1.20444778e-07, 2.61814180e-07, 5.92892012e-07, 8.02768170e-07, 8.96637346e-07, 1.10209706e-06, 1.30862656e-06, 1.56257097e-06, 1.92247392e-06, 2.46558848e-06])
- C2H6(pressure)float642.705e-26 9.492e-26 ... 1.366e-07
- units :
- v/v
array([2.70521508e-26, 9.49237903e-26, 2.75350094e-25, 7.16683450e-25, 1.71559477e-24, 3.85822616e-24, 8.33752520e-24, 1.76590988e-23, 3.70834639e-23, 7.73420076e-23, 1.60150136e-22, 3.26340753e-22, 6.51068144e-22, 1.27607845e-21, 2.46948946e-21, 4.75947226e-21, 9.13673260e-21, 1.74160246e-20, 3.29940929e-20, 6.22064161e-20, 1.16715044e-19, 2.18697327e-19, 4.10234850e-19, 7.68185388e-19, 1.42945835e-18, 2.61058621e-18, 4.62429653e-18, 7.84693352e-18, 1.26219536e-17, 2.03722803e-17, 3.38205200e-17, 5.49277989e-17, 8.29427545e-17, 1.14929281e-16, 1.54047597e-16, 2.03001962e-16, 2.61430752e-16, 3.30517620e-16, 4.14054040e-16, 5.10833758e-16, 6.00535504e-16, 6.70409164e-16, 7.32157485e-16, 8.21740893e-16, 9.45460830e-16, 1.08124167e-15, 1.26975398e-15, 1.61514121e-15, 2.21696295e-15, 3.25739763e-15, 4.88742230e-15, 7.34337552e-15, 1.10413445e-14, 1.66961965e-14, 2.56584530e-14, 4.08944781e-14, 6.86414555e-14, 1.21191242e-13, 2.22739928e-13, 4.13570347e-13, 7.87433884e-13, 1.57439379e-12, 3.34800184e-12, 7.58295597e-12, 1.55912079e-11, 3.29652048e-11, 7.02248864e-11, 1.49482265e-10, 3.17389577e-10, 5.05237113e-10, 7.87532243e-10, 1.23153998e-09, 1.94271464e-09, 2.84176670e-09, 3.32081591e-09, 3.84600471e-09, 4.65131402e-09, 5.99992712e-09, 8.29580046e-09, 1.18482278e-08, 1.91973042e-08, 3.22250663e-08, 5.83613957e-08, 1.04822679e-07, 1.08071740e-07, 9.55785387e-08, 9.64878165e-08, 9.73981862e-08, 1.02103085e-07, 1.13857073e-07, 1.36606822e-07])
- SiO(pressure)float643.205e-18 1.584e-18 ... 0.0001879
- units :
- v/v
array([3.20531602e-18, 1.58392284e-18, 1.04513120e-18, 8.13033281e-19, 7.18243694e-19, 6.97629332e-19, 7.19939629e-19, 7.65972718e-19, 8.25626122e-19, 8.99244674e-19, 9.88114784e-19, 1.11084580e-18, 1.28919470e-18, 1.53662884e-18, 1.86690529e-18, 2.28191902e-18, 2.80572012e-18, 3.48676038e-18, 4.37348575e-18, 5.52567101e-18, 7.02837708e-18, 8.95474534e-18, 1.13930983e-17, 1.45368050e-17, 1.87286024e-17, 2.46929968e-17, 3.41611416e-17, 5.05807829e-17, 8.14901475e-17, 1.30323160e-16, 1.96392005e-16, 3.05752366e-16, 5.30763586e-16, 1.04781241e-15, 2.16689138e-15, 4.55180579e-15, 1.00321730e-14, 2.30618192e-14, 5.27318883e-14, 1.22573835e-13, 3.02988341e-13, 8.16173587e-13, 2.29437521e-12, 6.14231440e-12, 1.56378500e-11, 4.01591028e-11, 1.00139617e-10, 2.22534049e-10, 4.37534599e-10, 7.75450174e-10, 1.32851005e-09, 2.29107058e-09, 3.96942191e-09, 6.75159320e-09, 1.09984859e-08, 1.70795610e-08, 2.49101446e-08, 3.41127448e-08, 4.37505514e-08, 5.26663888e-08, 6.04903831e-08, 6.55642190e-08, 6.54438683e-08, 5.94087711e-08, 4.83647946e-08, 3.78433553e-08, 2.93519606e-08, 2.29444187e-08, 1.82142369e-08, 1.43491874e-08, 1.16355934e-08, 9.86257232e-09, 8.89597602e-09, 8.81501698e-09, 9.79672050e-09, 1.21622271e-08, 1.71597908e-08, 2.80362183e-08, 5.40285067e-08, 1.26097765e-07, 3.51221713e-07, 1.20174489e-06, 4.97304304e-06, 2.97552902e-05, 1.17121766e-04, 1.93864775e-04, 1.93543687e-04, 1.93011144e-04, 1.92061146e-04, 1.90514020e-04, 1.87905132e-04])
- MgH(pressure)float641.098e-27 5.902e-28 ... 1.549e-05
- units :
- v/v
array([1.09844117e-27, 5.90172015e-28, 4.32579646e-28, 3.78404703e-28, 3.79462080e-28, 4.21333954e-28, 4.99296670e-28, 6.11390929e-28, 7.59191556e-28, 9.53330599e-28, 1.20903204e-27, 1.57186439e-27, 2.11477553e-27, 2.92807812e-27, 4.13844428e-27, 5.88733504e-27, 8.42875138e-27, 1.22065886e-26, 1.78550049e-26, 2.63219036e-26, 3.90852067e-26, 5.81424456e-26, 8.63609532e-26, 1.28668954e-25, 1.93713327e-25, 2.98997869e-25, 4.86013108e-25, 8.49872417e-25, 1.62742272e-24, 3.09956241e-24, 5.57308105e-24, 1.03825677e-23, 2.17792647e-23, 5.25587385e-23, 1.33428413e-22, 3.44597594e-22, 9.37625989e-22, 2.67046317e-21, 7.56710262e-21, 2.18342882e-20, 6.73799097e-20, 2.28330849e-19, 8.10465032e-19, 2.72832642e-18, 8.69726493e-18, 2.79880437e-17, 8.72080126e-17, 2.39900344e-16, 5.78880944e-16, 1.24693326e-15, 2.58818259e-15, 5.41030683e-15, 1.13744777e-14, 2.35216813e-14, 4.63996468e-14, 8.68284623e-14, 1.51617839e-13, 2.46982954e-13, 3.74327052e-13, 5.29525064e-13, 7.11479534e-13, 8.96738476e-13, 1.03222132e-12, 1.07045047e-12, 9.88107365e-13, 8.73189885e-13, 7.64207479e-13, 6.74543528e-13, 6.05492802e-13, 5.40450557e-13, 4.98002056e-13, 4.81705093e-13, 4.98785009e-13, 5.72057001e-13, 7.42571011e-13, 1.08806465e-12, 1.83539634e-12, 3.63926167e-12, 8.65200364e-12, 2.53174021e-11, 8.98518207e-11, 3.96845455e-10, 2.33236793e-09, 1.68692681e-08, 3.32153711e-07, 2.88636215e-06, 4.18557169e-06, 5.95143194e-06, 8.31771926e-06, 1.14331286e-05, 1.54918955e-05])
- OCS(pressure)float648.033e-09 8.056e-09 ... 7.51e-09
- units :
- v/v
array([8.03334622e-09, 8.05602540e-09, 8.05475412e-09, 8.04254546e-09, 8.02490547e-09, 8.00840943e-09, 7.99617479e-09, 7.98453927e-09, 7.97421516e-09, 7.96536620e-09, 7.94832488e-09, 7.92408564e-09, 7.89777244e-09, 7.86975768e-09, 7.84057282e-09, 7.79256270e-09, 7.73686477e-09, 7.68452961e-09, 7.63569329e-09, 7.59049851e-09, 7.54562090e-09, 7.50287279e-09, 7.46531581e-09, 7.43274082e-09, 7.40498323e-09, 7.38408967e-09, 7.36685671e-09, 7.35336579e-09, 7.34428538e-09, 7.33108713e-09, 7.29309786e-09, 7.25372524e-09, 7.20995821e-09, 7.16111387e-09, 7.10457987e-09, 7.03157103e-09, 6.97989616e-09, 6.95068139e-09, 6.91068282e-09, 6.86492547e-09, 6.80904571e-09, 6.75871080e-09, 6.71585912e-09, 6.67768351e-09, 6.63523899e-09, 6.59272078e-09, 6.55915937e-09, 6.53396278e-09, 6.51097063e-09, 6.48690471e-09, 6.46096551e-09, 6.44228167e-09, 6.42947182e-09, 6.41446568e-09, 6.38430465e-09, 6.35935179e-09, 6.34584540e-09, 6.34201322e-09, 6.33457787e-09, 6.28829082e-09, 6.23446445e-09, 6.19262211e-09, 6.15776083e-09, 6.12051175e-09, 5.84722538e-09, 5.58858672e-09, 5.34382276e-09, 5.11592321e-09, 4.90804320e-09, 4.22430874e-09, 3.63740227e-09, 3.17664659e-09, 2.83625363e-09, 2.47866848e-09, 1.95300436e-09, 1.58526995e-09, 1.37839732e-09, 1.31023490e-09, 1.37102047e-09, 1.52270163e-09, 1.89377759e-09, 2.40543493e-09, 3.18690165e-09, 4.04912452e-09, 4.65880769e-09, 5.13591519e-09, 5.52901840e-09, 5.87278685e-09, 6.26231251e-09, 6.77375207e-09, 7.50996692e-09])
- Li(pressure)float647.679e-12 6.305e-12 ... 6.115e-09
- units :
- v/v
array([7.67897569e-12, 6.30480646e-12, 5.60079462e-12, 5.22089545e-12, 5.06972633e-12, 5.06944131e-12, 5.15250121e-12, 5.31623010e-12, 5.53770287e-12, 5.81998659e-12, 5.94055156e-12, 5.93743070e-12, 6.00034468e-12, 6.12779414e-12, 6.31588067e-12, 6.45043504e-12, 6.58172547e-12, 6.78091071e-12, 7.05319116e-12, 7.40445431e-12, 7.74465260e-12, 8.09258656e-12, 8.53362107e-12, 9.09259648e-12, 9.81133132e-12, 1.06601703e-11, 1.18632980e-11, 1.36440003e-11, 1.63397288e-11, 1.91830290e-11, 2.04523694e-11, 2.20607435e-11, 2.45464219e-11, 2.83481854e-11, 3.32732540e-11, 3.90114732e-11, 4.70597397e-11, 5.83870351e-11, 6.45973529e-11, 6.96281862e-11, 7.44111331e-11, 8.01543443e-11, 8.65874118e-11, 1.03487892e-10, 1.29929342e-10, 1.64321383e-10, 2.08751507e-10, 2.58367006e-10, 3.05226112e-10, 3.49742992e-10, 3.96518015e-10, 4.51003173e-10, 5.15722301e-10, 5.92805281e-10, 6.69565166e-10, 7.44566532e-10, 8.12742590e-10, 8.71095443e-10, 9.22827456e-10, 9.60465530e-10, 9.84965515e-10, 9.93252810e-10, 9.78874538e-10, 9.39919202e-10, 8.65208186e-10, 7.88344631e-10, 7.16746877e-10, 6.53159336e-10, 5.97895868e-10, 5.33016289e-10, 4.78471910e-10, 4.35352113e-10, 4.03800890e-10, 3.84832008e-10, 3.76967583e-10, 3.80005338e-10, 3.96841811e-10, 4.31438555e-10, 4.90687102e-10, 5.90550304e-10, 7.50861622e-10, 1.02374266e-09, 1.49247798e-09, 2.25165289e-09, 3.05019608e-09, 3.77382469e-09, 4.52303551e-09, 5.10099204e-09, 5.53175030e-09, 5.85930190e-09, 6.11522386e-09])
- LiOH(pressure)float644.33e-10 4.522e-10 ... 2.3e-09
- units :
- v/v
array([4.32975589e-10, 4.52220822e-10, 4.73860448e-10, 4.99107243e-10, 5.29734491e-10, 5.64807549e-10, 6.02489600e-10, 6.47241731e-10, 6.99595343e-10, 7.60872212e-10, 8.01812489e-10, 8.22433870e-10, 8.45908730e-10, 8.73108613e-10, 9.04991844e-10, 9.28038260e-10, 9.49322939e-10, 9.77792967e-10, 1.01431958e-09, 1.05995402e-09, 1.10171690e-09, 1.14355316e-09, 1.19826093e-09, 1.26771013e-09, 1.35475895e-09, 1.44988076e-09, 1.56905010e-09, 1.72329550e-09, 1.92692378e-09, 2.11527024e-09, 2.14210327e-09, 2.17588033e-09, 2.21517338e-09, 2.26238026e-09, 2.32048669e-09, 2.37033106e-09, 2.45580742e-09, 2.58359367e-09, 2.99833738e-09, 3.52983346e-09, 4.11898386e-09, 4.82536720e-09, 5.65304112e-09, 5.59266820e-09, 5.08415731e-09, 4.64059186e-09, 4.29826199e-09, 4.08968630e-09, 3.97531418e-09, 3.88611144e-09, 3.80426442e-09, 3.72431657e-09, 3.66827410e-09, 3.71132971e-09, 3.74922629e-09, 3.78462275e-09, 3.82126358e-09, 3.86146229e-09, 3.96617258e-09, 4.11067933e-09, 4.26333470e-09, 4.41908030e-09, 4.58669341e-09, 4.77585869e-09, 5.04660838e-09, 5.34287486e-09, 5.65851759e-09, 5.98902814e-09, 6.33129748e-09, 6.66234597e-09, 7.00113756e-09, 7.34712767e-09, 7.69806495e-09, 7.98777629e-09, 8.11025987e-09, 8.20938670e-09, 8.30522269e-09, 8.40708784e-09, 8.51619887e-09, 8.59226662e-09, 8.66448743e-09, 8.56276679e-09, 8.09489267e-09, 7.10522119e-09, 6.38054826e-09, 5.72015808e-09, 4.73311324e-09, 3.89494550e-09, 3.21894408e-09, 2.69605130e-09, 2.30006222e-09])
- LiH(pressure)float642.283e-15 2.148e-15 ... 7.35e-09
- units :
- v/v
array([2.28333086e-15, 2.14826417e-15, 2.16292499e-15, 2.27088004e-15, 2.47167542e-15, 2.76031037e-15, 3.12611313e-15, 3.58984403e-15, 4.15979391e-15, 4.86141333e-15, 5.51564336e-15, 6.12212370e-15, 6.86254054e-15, 7.76559227e-15, 8.86233624e-15, 1.00195309e-14, 1.13147364e-14, 1.28961993e-14, 1.48345484e-14, 1.72177412e-14, 1.99051582e-14, 2.29880623e-14, 2.67931525e-14, 3.15505709e-14, 3.76110787e-14, 4.51044302e-14, 5.53009652e-14, 6.98908041e-14, 9.16811620e-14, 1.17921687e-13, 1.38016396e-13, 1.63219036e-13, 1.98277076e-13, 2.48760821e-13, 3.16618145e-13, 4.02279818e-13, 5.24938303e-13, 7.03440629e-13, 8.40588570e-13, 9.77938899e-13, 1.12526389e-12, 1.30079230e-12, 1.50559208e-12, 1.93157803e-12, 2.60804365e-12, 3.54602305e-12, 4.84885358e-12, 6.48857429e-12, 8.32630391e-12, 1.04050411e-11, 1.28821175e-11, 1.59972478e-11, 1.99689886e-11, 2.50724572e-11, 3.09814481e-11, 3.77624428e-11, 4.52955434e-11, 5.34824850e-11, 6.25756994e-11, 7.20869495e-11, 8.19701540e-11, 9.18701008e-11, 1.00957869e-10, 1.08494455e-10, 1.12097091e-10, 1.14820541e-10, 1.17395639e-10, 1.20273332e-10, 1.23709423e-10, 1.23815937e-10, 1.24637172e-10, 1.26960940e-10, 1.31530743e-10, 1.39563107e-10, 1.51665308e-10, 1.68900784e-10, 1.93869726e-10, 2.30299605e-10, 2.84321882e-10, 3.68952351e-10, 5.02511542e-10, 7.29714623e-10, 1.12547273e-09, 1.78346476e-09, 2.52202595e-09, 3.27651490e-09, 4.14701590e-09, 4.96491663e-09, 5.74485776e-09, 6.52715272e-09, 7.34986043e-09])
- LiCl(pressure)float646.089e-09 5.447e-09 ... 4.201e-11
- units :
- v/v
array([6.08914277e-09, 5.44650492e-09, 5.12947449e-09, 4.95731717e-09, 4.86850356e-09, 4.80162585e-09, 4.70593658e-09, 4.59329490e-09, 4.45402778e-09, 4.28792937e-09, 4.11940173e-09, 3.95963815e-09, 3.83513791e-09, 3.74291963e-09, 3.67931403e-09, 3.70818602e-09, 3.78537350e-09, 3.87028504e-09, 3.96273936e-09, 4.06222680e-09, 4.24168086e-09, 4.46336754e-09, 4.65606837e-09, 4.81715632e-09, 4.94520662e-09, 4.99524616e-09, 4.99989803e-09, 4.95997241e-09, 4.86883199e-09, 4.75730605e-09, 4.71238815e-09, 4.70633576e-09, 4.79010026e-09, 4.98838114e-09, 5.29032452e-09, 5.68871109e-09, 6.11574328e-09, 6.56682467e-09, 6.35347833e-09, 6.01990980e-09, 5.61786561e-09, 5.24078908e-09, 4.88894083e-09, 4.95784127e-09, 5.27790685e-09, 5.67960639e-09, 6.20799057e-09, 6.62773148e-09, 6.65646264e-09, 6.68251617e-09, 6.70635904e-09, 6.80825354e-09, 6.92333762e-09, 6.77239619e-09, 6.60646987e-09, 6.47941933e-09, 6.40742531e-09, 6.37977496e-09, 6.20883064e-09, 5.96178129e-09, 5.73457251e-09, 5.55846594e-09, 5.42120868e-09, 5.30516724e-09, 5.01768642e-09, 4.74959885e-09, 4.49824940e-09, 4.26502057e-09, 4.05181883e-09, 3.69468180e-09, 3.37193993e-09, 3.09697168e-09, 2.87179008e-09, 2.69384235e-09, 2.52091650e-09, 2.34103128e-09, 2.20245585e-09, 2.10637902e-09, 2.02912637e-09, 1.90119496e-09, 1.81276314e-09, 1.66523238e-09, 1.47794659e-09, 1.19654430e-09, 7.57295950e-10, 4.54187180e-10, 2.78483046e-10, 1.68454891e-10, 1.02886721e-10, 6.46025004e-11, 4.20081358e-11])
- transit_depth(wavelength)float640.02463 0.02476 ... 0.02521 0.02521
- units :
- (R_jup/R_jup)**2
array([0.02462922, 0.024757 , 0.02481346, ..., 0.0252136 , 0.02521373, 0.02521385])
- pressurePandasIndex
PandasIndex(Index([ 1e-06, 1.2671743143250784e-06, 1.6057307428852327e-06, 2.0347407531062896e-06, 2.578371218646756e-06, 3.26724578106422e-06, 4.140169932351558e-06, 5.246316995216892e-06, 6.647998141145957e-06, 8.424172486141026e-06, 1.0674894993881945e-05, 1.3526952744364565e-05, 1.7141007068747908e-05, 2.1720643879181908e-05, 2.752384201430155e-05, 3.487750563206435e-05, 4.41958792846802e-05, 5.6003883028558584e-05, 7.096668207625546e-05, 8.992715669990485e-05, 0.00011395338313040582, 0.00014439880013329483, 0.0001829784505482719, 0.00023186559260977168, 0.00029381412333086515, 0.000372313710270813, 0.00047178637052624296, 0.0005978355705795093, 0.0007575618792282317, 0.0009599629548698516, 0.0012164403991146804, 0.0015414420286654685, 0.0019532757457460226, 0.0024751408538035225, 0.003136434914276465, 0.0039744097619235155, 0.005036269964912324, 0.006381831939543761, 0.00808689351212925, 0.0102475037412523, 0.012985373526865058, 0.016454731795160254, 0.020851013479935218, 0.02642186870941988, 0.03348111336504638, 0.04242640687119287, 0.053761653036280624, 0.06812538582323154, 0.08632673906868484, 0.10939102638728067, 0.13861749885561894, 0.1756525340658263, 0.22258237941432546, 0.2820506740151923, 0.3574073694501275, 0.4528974383176954, 0.5739000008598101, 0.7272313400806903, 0.9215288747224568, 1.16773771995719, 1.4797272445982832, 1.875072356561967, 2.3760435277363143, 3.0108613280658054, 3.8152861389196815, 4.834632597039524, 6.1263222461672315, 7.7631181916214205, 9.837223971492415, 12.465477540938124, 15.795932955672937, 20.016200512229762, 25.364015159477983, 32.14062851824241, 40.72777890458089, 51.609195307395666, 65.39784667651817, 82.87047152065334, 105.01133292697985, 133.06766379810796, 168.61992563220787, 213.67083864453826, 270.7581984506578, 343.0978344696051, 434.7647631404402, 550.9227406251937, 698.1151460978214, 884.6335815764605, 1120.9849521630872, 1420.4833381259884, 1799.9999999999998], dtype='float64', name='pressure'))
- wavelengthPandasIndex
PandasIndex(Index([ 5.999504507075861, 5.997505005490434, 5.99550617029447, 5.993508001265877, 5.9915104981826355, 5.989513660822802, 5.987517488964505, 5.985521982385947, 5.983527140865405, 5.981532964181231, ... 0.3009525047579417, 0.3008522039731532, 0.3007519366163883, 0.3006517026765062, 0.3005515021423698, 0.3004513350028456, 0.300351201246804, 0.300251100863119, 0.30015103384066844, 0.30005100016833364], dtype='float64', name='wavelength', length=8988))
- pressure_cldPandasIndex
PandasIndex(Index([ 1.1183063e-06, 1.38291166e-06, 1.71012598e-06, 2.11476333e-06, 2.61514298e-06, 3.23391875e-06, 3.99910467e-06, 4.94534321e-06, 6.11547372e-06, 7.56247184e-06, 9.35184796e-06, 1.1564613e-05, 1.43009461e-05, 1.76847301e-05, 2.18691601e-05, 2.70436789e-05, 3.3442554e-05, 4.13554836e-05, 5.1140712e-05, 6.32412488e-05, 7.82049249e-05, 9.67091954e-05, 0.000119591809, 0.000147888738, 0.000182881077, 0.000226153043, 0.000279663703, 0.000345835658, 0.000427664731, 0.000528855595, 0.000653989491, 0.000808731644, 0.00100008774, 0.0012367211, 0.00152934489, 0.00189120716, 0.0023386906, 0.00289205425, 0.00357635073, 0.00442256036, 0.00546899386, 0.00676302671, 0.00836324403, 0.0103420929, 0.0127891624, 0.0158152394, 0.0195573242, 0.0241848335, 0.0299072698, 0.0369837066, 0.0457345175, 0.0565558806, 0.0699377144, 0.0864858587, 0.106949502, 0.132255102, 0.163548325, 0.20224592, 0.250099852, 0.309276628, 0.382455374, 0.472949134, 0.584854858, 0.723238885, 0.894366317, 1.10598465, 1.36767456, 1.69128359, 2.09146259, 2.58632899, 3.19828701, 3.95504201, 4.89085478, 6.04809263, 7.47914755, 9.24880808, 11.4371926, 14.1433764, 17.4898775, 21.6282028, 26.7457081, 33.0740796, 40.8998235, 50.577237, 62.5444485, 77.3432529, 95.6436409, 118.274131, 146.259281, 180.866069], dtype='float64', name='pressure_cld'))
- wno_cldPandasIndex
PandasIndex(Index([ 43.95005515731922, 72.04974314266569, 102.29969719289632, 135.6999402920263, 185.60106906215782, 236.8994598692315, 279.6968086594132, 336.7003367003367, 402.592696968477, 467.3989249824725, ... 21505.37634408602, 22471.91011235955, 23474.17840375587, 24509.80392156863, 25839.793281653747, 27624.309392265193, 29673.590504451036, 32051.28205128205, 34843.20557491289, 37313.432835820895], dtype='float64', name='wno_cld', length=196))
- author :
- Sarah E. Moran
- contact :
- semoran@lpl.arizona.edu
- code :
- {"spectrum": "PICASO", "climate": "PICASO", "chemistry": "PICASO", "clouds": "Virga"}
- doi :
- Mukherjee et al., submitted; Batalha et al., in prep
- planet_params :
- {"rp": {"value": 1.279, "unit": "jupiterRad"}, "mp": {"value": 0.28, "unit": "jupiterMass"}, "tint": 100.0, "heat_redis": 0.5, "mh": 3.162, "cto": 0.458, "rainout": "None", "p_quench": "None", "p_reference": {"value": 10.0, "unit": "bar"}, "logkzz": {"value": 9.0, "unit": "cm2 / s"}}
- stellar_params :
- {"rs": {"value": 0.932, "unit": "solRad"}, "logg": 4.38933, "steff": 5326.6, "feh": -0.03, "ms": {"value": 0.913, "unit": "solMass"}}
- orbit_params :
- {"sma": 0.0486}
- atmo_params :
- {"mh": 0.5, "cto": 1.0}
- cld_params :
- {"fsed": 0.6, "species": "Na2S, MnS, MgSiO3"}
[20]:
opa = jdi.opannection(wave_range=[3,5])
case = jdi.input_xarray(xr_usr, opa)
#if you need to rerun your spectrum
#out = case.spectrum(opa,calculation='transmisson')
See Contribution From Each Molecule¶
One of the most common plots that was also used in the original paper is the “leave one out” method to see how each molecule is affecting our spectrum.
[21]:
#copy atmo before modifying and rerunning picaso
og_atmo = jdi.copy.deepcopy(case.inputs['atmosphere']['profile'])
#atmo
w,f,l =[],[],[]
for iex in ['CH4','H2O','CO2',None]:
case.atmosphere(df = og_atmo,exclude_mol=iex, delim_whitespace=True)
df= case.spectrum(opa, full_output=True,calculation='transmission') #note the new last key
wno, rprs2 = df['wavenumber'] , df['transit_depth']
wno, rprs2 = jdi.mean_regrid(wno, rprs2, R=150)
w +=[wno]
f+=[rprs2]
if iex==None:
leg='all'
else:
leg = f'No {iex}'
l+=[leg]
jpi.show(jpi.spectrum(w,f,legend=l))
Quantify Molecular Detection using Guassian Fitting¶
For very gaussian shaped molecules (like CO2 in this case), we can use a simple Gaussian fitting technique to quantify the significance of our detection. Note this ONLY works in cases where the shape of the molecule is gaussian with a single peak and well-shaped wings.
[22]:
#grab file to test
top_model_file = np.array(out['list_of_files']['picaso_cldy']
)[out['rank_order']['picaso_cldy']['firefly']][0]
min_wave = 3 #min wave to search for gauss peak
max_wave = 5 #max wave to search for gauss peak
out = lyz.detection_test(fitter,'CO2',min_wave,max_wave,'picaso_cldy','firefly',
top_model_file,
#opa_kwargs={wave_range=[]}#this is where you input arguments for opannection
plot=True)
8676it [00:16, 535.41it/s, +500 | bound: 26 | nc: 1 | ncall: 37091 | eff(%): 25.077 | loglstar: -inf < -77.771 < inf | logz: -94.391 +/- 0.182 | dlogz: 0.001 > 0.509]
2562it [00:06, 389.20it/s, +500 | bound: 2 | nc: 1 | ncall: 19385 | eff(%): 16.214 | loglstar: -inf < -466.966 < inf | logz: -471.182 +/- 0.073 | dlogz: 0.001 > 0.509]
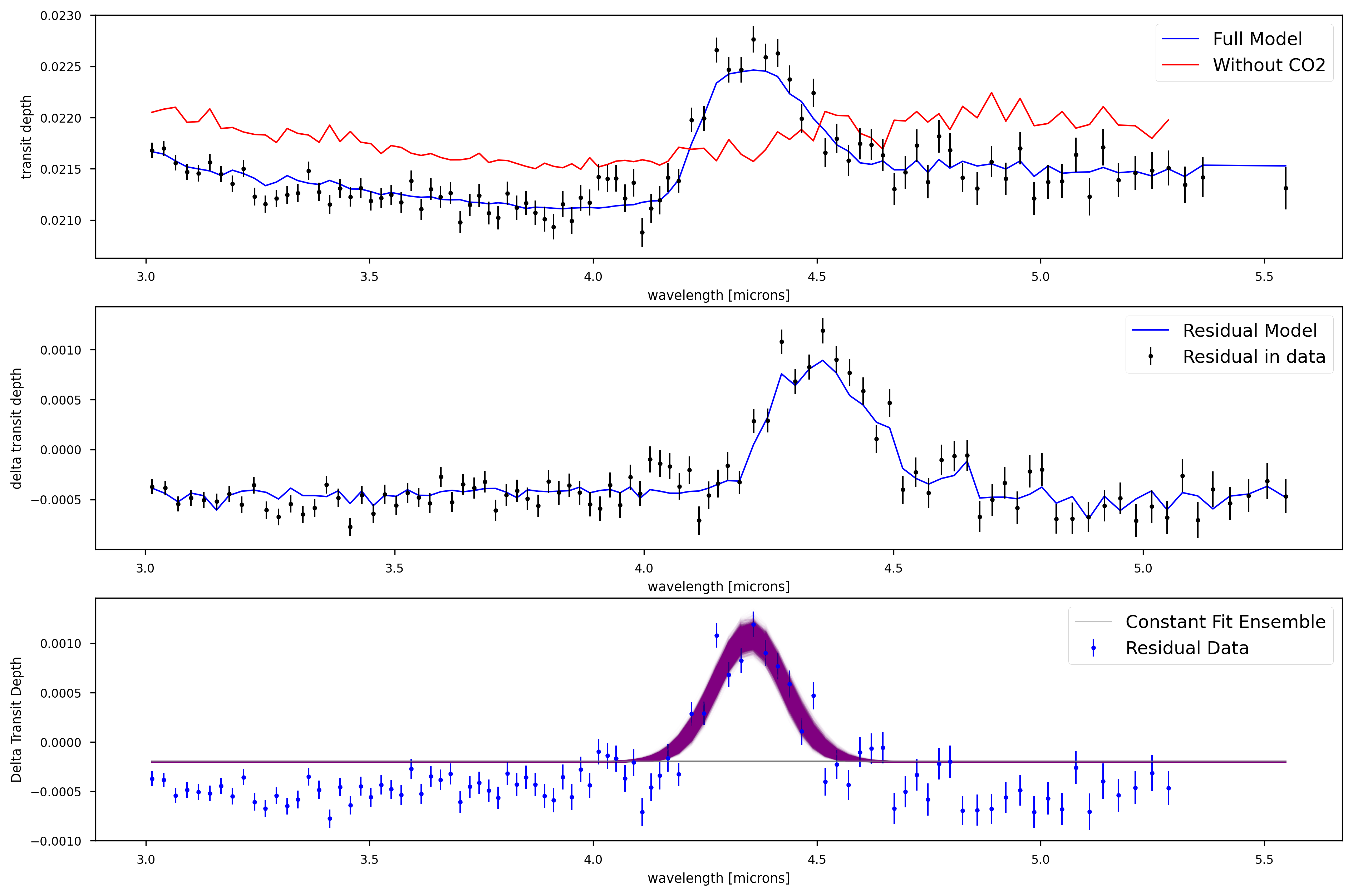
By comparing the line fit to the single Gaussian fit, we can use the methodology of Trotta 2008 to get out a sigma detection significance. In this case we can see that the single gaussian fit is preferred over the line model at 26 sigma.
[23]:
out['sigma_single_v_line']
[23]:
27.575580879765575